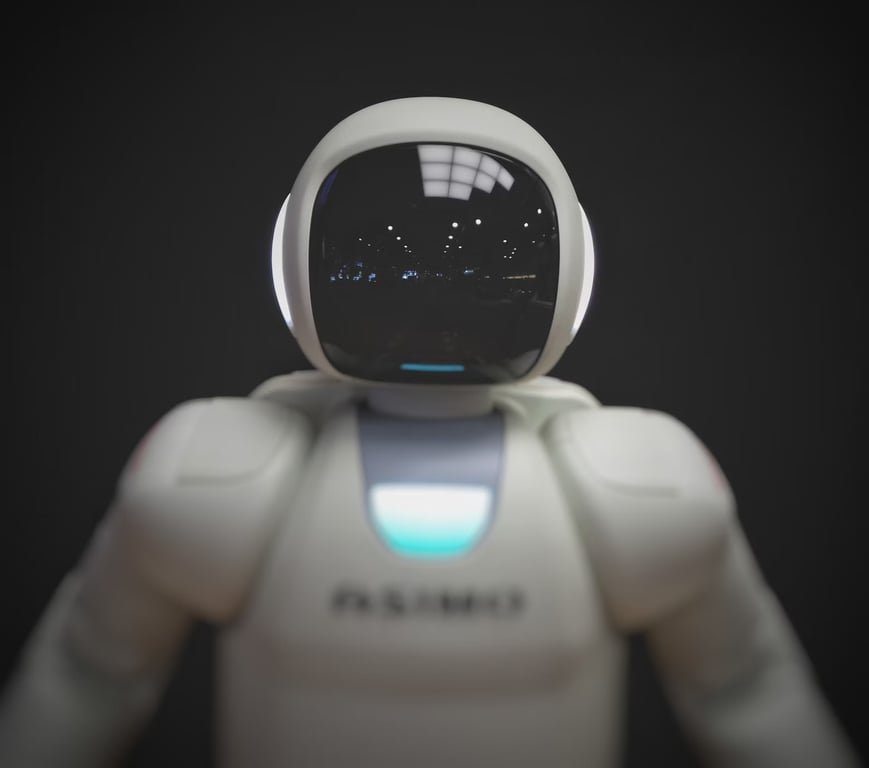
Photo by Possessed Photography on Unsplash
Time series analysis is a statistical method used to analyze time-ordered data points. It plays a crucial role in various domains, including finance, healthcare, energy, and meteorology. Two of the most popular techniques for time series forecasting are ARIMA (AutoRegressive Integrated Moving Average) and LSTM (Long Short-Term Memory) models. This blog provides a beginner-friendly introduction to these techniques, their applications, and Python implementation examples.
Table of Contents
What is Time Series Analysis?
Applications of Time Series Analysis
Understanding ARIMA Models
3.1. Components of ARIMA
3.2. Steps to Build an ARIMA Model
3.3. ARIMA Implementation in Python
Introduction to LSTM Models
4.1. Why LSTM for Time Series?
4.2. Structure of an LSTM Network
4.3. LSTM Implementation in Python
Comparing ARIMA and LSTM Models
Choosing the Right Model for Your Use Case
Conclusion
1. What is Time Series Analysis?
Time series analysis involves analyzing data points collected or recorded at specific intervals over time. The primary goal is to understand underlying patterns, detect trends, and make forecasts.
Characteristics of Time Series Data
Trend: The long-term movement in the data.
Seasonality: Regular, periodic fluctuations.
Noise: Random variations in the data.
Example
A stock price dataset showing daily closing prices is a typical time series.
2. Applications of Time Series Analysis
Finance: Forecasting stock prices, exchange rates.
Healthcare: Predicting patient admissions.
Energy: Modeling electricity demand.
Weather: Forecasting temperatures and rainfall.
3. Understanding ARIMA Models
3.1. Components of ARIMA
ARIMA stands for:
AutoRegressive (AR): Uses past values to predict the current value.
Integrated (I): Makes the series stationary by differencing.
Moving Average (MA): Models the relationship between past errors and current values.
ARIMA is denoted as ARIMA(p, d, q), where:
p: Order of the AR term.
d: Number of differences needed to make the series stationary.
q: Order of the MA term.
3.2. Steps to Build an ARIMA Model
Data Preprocessing:
Remove missing values.
Convert the data to a stationary series.
Parameter Selection:
Use techniques like ACF (Autocorrelation Function) and PACF (Partial Autocorrelation Function).
Model Fitting:
Fit the ARIMA model with the chosen parameters.
Model Evaluation:
Check residuals for randomness and calculate error metrics.
3.3. ARIMA Implementation in Python
import pandas as pd
from statsmodels.tsa.arima.model import ARIMA
import matplotlib.pyplot as plt
# Load the dataset
data = pd.read_csv('timeseries.csv', index_col='Date', parse_dates=True)
# Make the series stationary
data_diff = data.diff().dropna()
# Fit the ARIMA model
model = ARIMA(data_diff, order=(1, 1, 1))
model_fit = model.fit()
# Forecast
forecast = model_fit.forecast(steps=10)
# Plot results
plt.plot(data, label="Original Data")
plt.plot(forecast, label="Forecast", color='red')
plt.legend()
plt.show()
4. Introduction to LSTM Models
4.1. Why LSTM for Time Series?
LSTMs are a type of recurrent neural network (RNN) designed to handle sequential data. Unlike traditional RNNs, LSTMs can learn long-term dependencies, making them ideal for time series forecasting.
4.2. Structure of an LSTM Network
An LSTM network consists of:
Input Layer: Processes time series data.
Hidden Layers: Contains LSTM units to retain memory over time.
Output Layer: Predicts the next value in the sequence.
4.3. LSTM Implementation in Python
import numpy as np
import pandas as pd
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import LSTM, Dense
from sklearn.preprocessing import MinMaxScaler
# Load and preprocess data
data = pd.read_csv('timeseries.csv', index_col='Date', parse_dates=True)
scaler = MinMaxScaler()
scaled_data = scaler.fit_transform(data)
# Prepare data for LSTM
def create_dataset(data, look_back=1):
X, y = [], []
for i in range(len(data) - look_back):
X.append(data[i:(i + look_back), 0])
y.append(data[i + look_back, 0])
return np.array(X), np.array(y)
look_back = 3
X, y = create_dataset(scaled_data, look_back)
X = X.reshape((X.shape[0], X.shape[1], 1))
# Build the LSTM model
model = Sequential([
LSTM(50, activation='relu', input_shape=(look_back, 1)),
Dense(1)
])
model.compile(optimizer='adam', loss='mse')
# Train the model
model.fit(X, y, epochs=20, batch_size=32, verbose=1)
# Make predictions
predictions = model.predict(X)
# Plot predictions
import matplotlib.pyplot as plt
plt.plot(scaled_data, label='Original Data')
plt.plot(np.arange(look_back, len(predictions) + look_back), predictions, label='Predictions', color='red')
plt.legend()
plt.show()
5. Comparing ARIMA and LSTM Models
ARIMA
Data Type : Univariate
Trend : Handles trends explicitly
Feature : Statistical approach
Scalability : Limited to small datasets
LSTM
Data Type : Univariate/Multivariate
Trend : Learns trends implicitly
Feature : Deep learning approach
Scalability : Handles large datasets
6. Choosing the Right Model for Your Use Case
ARIMA: Best for smaller datasets with clear seasonality and trend patterns.
LSTM: Suitable for larger datasets and complex, nonlinear patterns.
7. Conclusion
Time series analysis is a powerful tool for forecasting and decision-making. ARIMA models are reliable and statistically robust for small datasets, while LSTMs excel at capturing complex relationships in larger datasets. Both models have their strengths, and choosing the right one depends on your data and objectives. Start experimenting with these techniques in Python to unlock the potential of your time series data.
Happy coding!