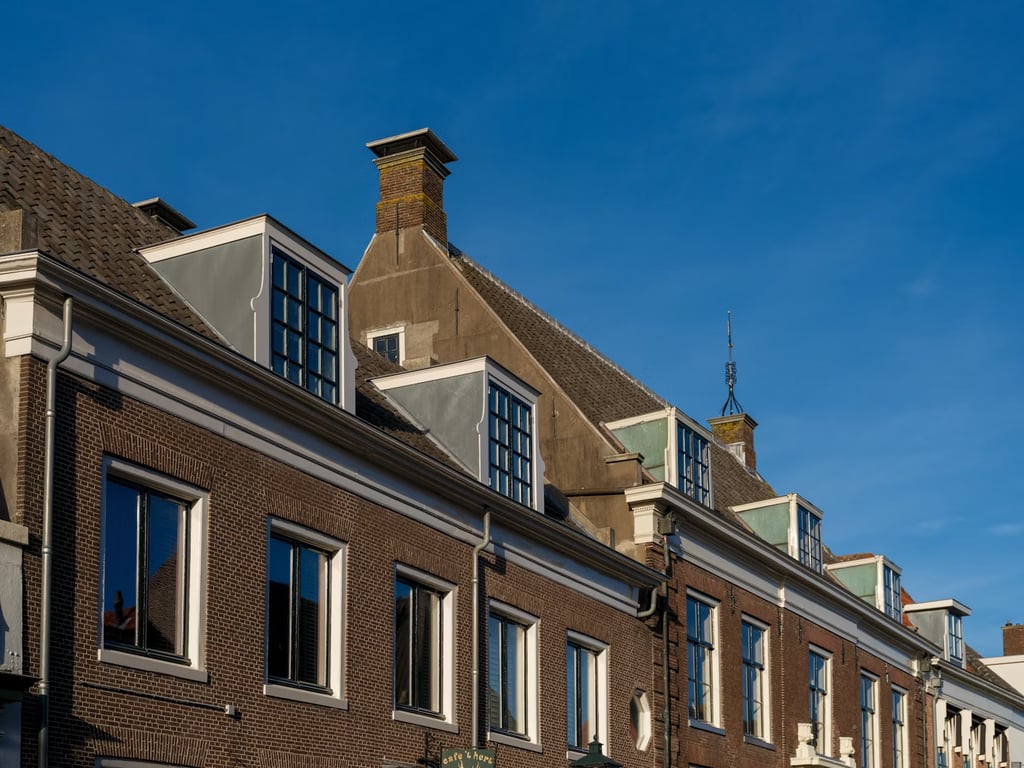
Photo by Haberdoedas on Unsplash
Machine Learning (ML) has rapidly become one of the most influential technologies of the 21st century. From self-driving cars to personalized recommendations, ML algorithms are transforming industries across the globe. If you're a Python enthusiast or a beginner looking to break into the field of machine learning, then Python and its associated libraries—specifically Scikit-Learn—are powerful tools you should familiarize yourself with.
In this blog, we’ll provide a step-by-step introduction to machine learning with Python and Scikit-Learn, one of the most popular and user-friendly libraries for implementing machine learning algorithms. You’ll learn what machine learning is, how to set up your Python environment, and how to implement basic machine learning tasks using Scikit-Learn.
1. What is Machine Learning?
Machine Learning refers to a branch of artificial intelligence (AI) that enables computers to learn from data and make decisions or predictions without being explicitly programmed. Rather than programming a computer with specific instructions for every task, ML algorithms allow the system to recognize patterns in data and improve its performance over time.
Key Components of Machine Learning:
Data: ML algorithms require data to learn patterns and make predictions.
Model: A mathematical representation of a real-world process that learns from the data.
Training: The process where the model learns from the data.
Prediction: Once the model has been trained, it can be used to make predictions on new data.
2. Why Use Python for Machine Learning?
Python has become the go-to language for machine learning due to its simplicity, readability, and the wealth of libraries available for data analysis and machine learning. Some reasons why Python is favored for ML include:
Easy Syntax: Python’s syntax is clear and readable, making it ideal for rapid development and experimentation.
Libraries: Python offers several libraries like NumPy, Pandas, Matplotlib, and Scikit-Learn that simplify tasks like data manipulation, visualization, and machine learning.
Community Support: The Python community is vast, with active discussions, tutorials, and resources for ML practitioners of all skill levels.
Cross-Platform Compatibility: Python runs seamlessly on different operating systems, making it easy to develop and deploy machine learning models.
3. Installing and Setting Up Scikit-Learn
Before diving into Scikit-Learn, you'll need to install it. You can easily install Scikit-Learn using pip (Python's package manager). Open your terminal or command prompt and run:
pip install scikit-learn
Additionally, you’ll want to install NumPy, Pandas, and Matplotlib for data manipulation and visualization:
pip install numpy pandas matplotlib
After installation, you can import Scikit-Learn and start using it in your Python script:
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
4. Overview of Scikit-Learn
Scikit-Learn is a versatile library for machine learning in Python that provides simple and efficient tools for data analysis and predictive modeling. Some key features of Scikit-Learn include:
Classification: Identifying which category an object belongs to (e.g., spam vs. non-spam emails).
Regression: Predicting a continuous-valued attribute associated with an object (e.g., predicting house prices).
Clustering: Grouping similar objects together (e.g., customer segmentation).
Dimensionality Reduction: Reducing the number of features or variables in a dataset (e.g., PCA).
Model Selection: Choosing the best model or algorithm for a given problem.
5. Types of Machine Learning Algorithms
Machine learning algorithms are typically divided into three main types based on the learning process:
5.1. Supervised Learning
In supervised learning, the algorithm learns from labeled data, meaning the output (target) for each input (feature) is already known. The goal is to learn the mapping between inputs and outputs so that the model can predict outputs for new, unseen inputs. Examples include linear regression and decision trees.
5.2. Unsupervised Learning
In unsupervised learning, the algorithm works with unlabeled data. The goal is to find hidden patterns or relationships within the data. Clustering algorithms like k-means and dimensionality reduction techniques like Principal Component Analysis (PCA) are examples of unsupervised learning.
5.3. Reinforcement Learning
In reinforcement learning, an agent learns by interacting with its environment and receiving feedback (rewards or punishments) based on its actions. It’s used in applications such as robotics, gaming, and autonomous driving.
6. Building a Machine Learning Model with Scikit-Learn
Let’s walk through the steps involved in building a machine learning model using Scikit-Learn.
6.1. Step 1: Loading the Dataset
You can load data from a variety of sources, such as CSV files, Excel files, or databases. Scikit-Learn also provides a set of built-in datasets for practice. Let’s load the Iris dataset (a popular dataset for classification problems).
from sklearn.datasets import load_iris
iris = load_iris()
X = iris.data # Features
y = iris.target # Labels
6.2. Step 2: Preprocessing Data
Before using data to train a machine learning model, you may need to preprocess it. This can include handling missing values, encoding categorical variables, and scaling features. Scikit-Learn provides tools like StandardScaler to scale data.
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
6.3. Step 3: Splitting Data into Training and Testing Sets
To evaluate how well your model generalizes to new data, you should split your dataset into a training set (to train the model) and a testing set (to evaluate the model's performance).
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X_scaled, y, test_size=0.3, random_state=42)
6.4. Step 4: Training a Model
Now it’s time to train your model. For this example, we'll use the Random Forest Classifier—a versatile algorithm for classification tasks.
from sklearn.ensemble import RandomForestClassifier
clf = RandomForestClassifier()
clf.fit(X_train, y_train)
6.5. Step 5: Making Predictions
Once the model is trained, you can make predictions on the test data.
y_pred = clf.predict(X_test)
6.6. Step 6: Evaluating the Model
Finally, you can evaluate the model’s performance using metrics like accuracy, precision, and recall. Here, we'll compute the accuracy of the Random Forest model.
from sklearn.metrics import accuracy_score
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy * 100:.2f}%")
7. Common Algorithms in Scikit-Learn
Here are a few common algorithms you can implement with Scikit-Learn:
7.1. Linear Regression
Linear regression is used to predict a continuous target variable based on one or more features.
from sklearn.linear_model import LinearRegression
model = LinearRegression()
model.fit(X_train, y_train)
7.2. Decision Trees
Decision trees are used for both classification and regression tasks. They work by recursively splitting the dataset into subsets based on feature values.
from sklearn.tree import DecisionTreeClassifier
model = DecisionTreeClassifier()
model.fit(X_train, y_train)
7.3. k-Nearest Neighbors (k-NN)
k-NN is a simple classification algorithm that classifies new data points based on the majority label of the nearest neighbors.
from sklearn.neighbors import KNeighborsClassifier
model = KNeighborsClassifier(n_neighbors=3)
model.fit(X_train, y_train)
7.4. Support Vector Machines (SVM)
SVM is a powerful classification algorithm that works well for both linear and non-linear decision boundaries.
from sklearn.svm import SVC
model = SVC()
model.fit(X_train, y_train)
8. Conclusion
Machine learning is an exciting and growing field, and Python—along with Scikit-Learn—provides an excellent platform for getting started. In this blog, we’ve learned how to use Scikit-Learn to build machine learning models, from loading and preprocessing data to training a model and evaluating its performance.
With a solid understanding of the basics, you’re well-equipped to start exploring more advanced topics and algorithms in machine learning. Continue experimenting, learning, and improving your skills, and you’ll soon be able to tackle more complex machine learning challenges.
Happy coding!