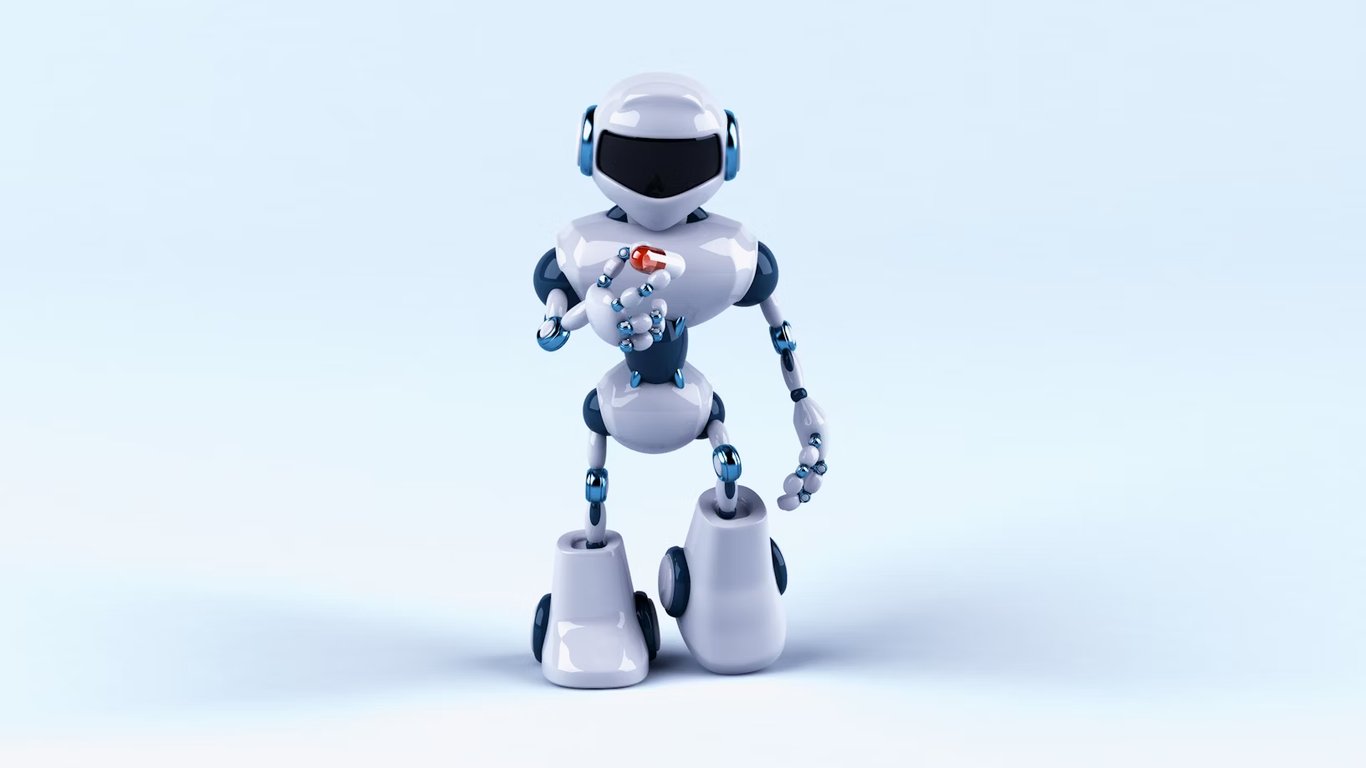
Photo by julien Tromeur on Unsplash
Data cleaning and preprocessing are essential steps in the data science workflow. Before building models or conducting any analysis, it's crucial to ensure that your data is clean, consistent, and ready for use. In this blog, we’ll explore various techniques and Python tools that can help data scientists clean and preprocess their datasets efficiently. Whether you are working with structured, unstructured, or semi-structured data, the tips provided here will guide you in improving your data quality.
Table of Contents
Understanding Data Cleaning and Preprocessing
Common Data Quality Issues
Key Steps in Data Cleaning and Preprocessing
3.1. Handling Missing Values
3.2. Removing Duplicates
3.3. Data Normalization and Scaling
3.4. Encoding Categorical Variables
3.5. Handling Outliers
3.6. Feature Engineering
Tools for Data Cleaning in Python
4.1. Pandas
4.2. NumPy
4.3. Scikit-learn
4.4. OpenRefine
Best Practices for Data Preprocessing
Conclusion
1. Understanding Data Cleaning and Preprocessing
Data cleaning and preprocessing refer to the process of preparing raw data for analysis or machine learning tasks. It involves identifying and correcting errors, handling missing values, standardizing formats, and transforming data into a usable form.
Why is this important?
Accuracy: Clean data ensures that the results of your analysis are accurate.
Model Performance: Machine learning models perform better on well-prepared data.
Consistency: Preprocessing ensures that your data is in the correct format and structure.
2. Common Data Quality Issues
Before diving into the cleaning techniques, let’s take a look at some common data quality issues:
Missing Values: Sometimes, data is missing from a dataset, which can affect analysis or models.
Duplicate Data: Duplicate rows in a dataset can lead to bias and incorrect conclusions.
Incorrect Data Types: For instance, numerical data might be mistakenly represented as strings.
Outliers: Extreme values can distort statistical analysis and models.
Inconsistent Data: Variations in the formatting, spelling, or units of data can make it difficult to analyze.
3. Key Steps in Data Cleaning and Preprocessing
3.1. Handling Missing Values
Missing data is a common issue in real-world datasets. There are several strategies to handle missing values:
Removing Missing Data: If the number of missing values is small, you can drop rows or columns with missing data.
Imputation: You can replace missing values with:
The mean, median, or mode of the column (for numerical data).
The most frequent value (for categorical data).
Forward/Backward Fill: For time-series data, you can use the previous or next available value to fill in the gaps.
import pandas as pd
# Load dataset
data = pd.read_csv('data.csv')
# Drop rows with missing values
data_clean = data.dropna()
# Fill missing values with the mean of the column
data_clean = data.fillna(data.mean())
3.2. Removing Duplicates
Duplicate records can skew analysis and machine learning models. It's important to identify and remove them.
Example in Python:# Remove duplicate rows based on all columns
data_clean = data.drop_duplicates()
# Remove duplicates based on specific columns
data_clean = data.drop_duplicates(subset=['Column1', 'Column2'])
3.3. Data Normalization and Scaling
In machine learning, algorithms like K-nearest neighbors (KNN) or gradient descent are sensitive to the scale of data. Normalization (scaling data to a specific range) or standardization (scaling data to have zero mean and unit variance) is often necessary.
Normalization: Scales values to a [0, 1] range.
Standardization: Centers values around zero with a standard deviation of one.
from sklearn.preprocessing import MinMaxScaler
scaler = MinMaxScaler()
data_scaled = scaler.fit_transform(data[['Column1', 'Column2']])
Example of Standardization:
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
data_standardized = scaler.fit_transform(data[['Column1', 'Column2']])
3.4. Encoding Categorical Variables
Many machine learning algorithms only accept numerical input, so categorical variables (e.g., 'Gender', 'City') need to be converted into numerical format. Common techniques include:
Label Encoding: Converts categories into numeric labels.
One-Hot Encoding: Creates new binary columns for each category.
# One-hot encode categorical columns
data_encoded = pd.get_dummies(data, columns=['CategoryColumn'])
Example of Label Encoding using Scikit-learn:
from sklearn.preprocessing import LabelEncoder
encoder = LabelEncoder()
data['CategoryEncoded'] = encoder.fit_transform(data['CategoryColumn'])
3.5. Handling Outliers
Outliers can distort statistical analyses and machine learning models. There are several ways to handle them:
Z-Score Method: Identify outliers by calculating the Z-score for each data point. Values greater than a threshold (e.g., 3) are considered outliers.
Interquartile Range (IQR): Outliers are defined as values outside the range of the first quartile (Q1) and third quartile (Q3).
from scipy import stats
z_scores = stats.zscore(data[['Column1', 'Column2']])
data_no_outliers = data[(z_scores < 3).all(axis=1)]
Example using IQR:Q1 = data[['Column1', 'Column2']].quantile(0.25)
Q3 = data[['Column1', 'Column2']].quantile(0.75)
IQR = Q3 - Q1
data_no_outliers = data[~((data[['Column1', 'Column2']] < (Q1 - 1.5 * IQR)) | (data[['Column1', 'Column2']] > (Q3 + 1.5 * IQR))).any(axis=1)]
3.6. Feature Engineering
Feature engineering is the process of transforming raw data into features that better represent the underlying patterns to the machine learning algorithms. It includes:
Creating new features: Combine existing features to create new, informative ones.
Polynomial Features: Generate polynomial features to capture interactions between variables.
Log Transformation: Apply a logarithmic transformation to reduce skewness in data.
from sklearn.preprocessing import PolynomialFeatures
poly = PolynomialFeatures(degree=2)
data_poly = poly.fit_transform(data[['Column1', 'Column2']])
4. Tools for Data Cleaning in Python
4.1. Pandas
Pandas is the most widely used library for data manipulation and cleaning. It provides easy-to-use data structures such as DataFrames and Series to handle and preprocess data.
4.2. NumPy
NumPy is useful for handling numerical data, performing mathematical operations, and working with arrays.
4.3. Scikit-learn
Scikit-learn is great for preprocessing tasks like scaling, encoding, and splitting datasets. It also provides tools for feature selection and dimensionality reduction.
4.4. OpenRefine
While not a Python library, OpenRefine is a powerful tool for data cleaning that can handle large datasets with messy data.
5. Best Practices for Data Preprocessing
Understand Your Data: Before cleaning, spend time exploring and understanding the data.
Document Your Process: Keep track of the steps you’ve taken, as data cleaning can involve many iterative steps.
Avoid Over-Cleaning: Be cautious of removing too much data; excessive cleaning might result in losing valuable information.
Use Visualizations: Visualizations can help identify outliers, missing values, and other issues.
Automate Repetitive Tasks: If you're working with similar datasets often, automate repetitive tasks using functions or scripts.
6. Conclusion
Data cleaning and preprocessing are the foundation of any data science or machine learning project. By following the best practices and using Python libraries like Pandas, NumPy, and Scikit-learn, data scientists can efficiently clean and preprocess their datasets. With clean data, you can build accurate models and gain insights that lead to data-driven decisions. Make sure to apply these techniques to your datasets, and you’ll be on your way to mastering data preprocessing.
Happy coding!