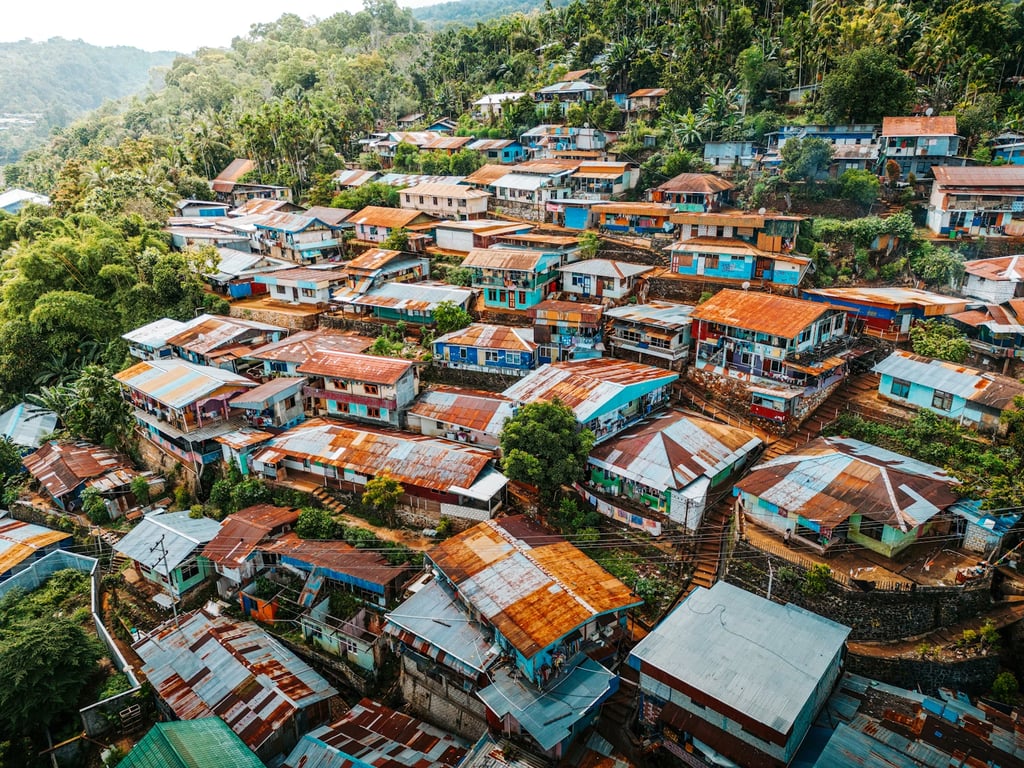
Photo by Simon Spring on Unsplash
Data automation is a critical component in today's data-driven world. Whether you're handling large datasets, performing repetitive tasks, or building data pipelines, automation can save you time, reduce errors, and ensure more efficient workflows. Python, with its simplicity and powerful libraries, is one of the most popular languages for automating data tasks. When combined with Jupyter Notebooks, which offer an interactive environment for writing and running Python code, the power of automation is greatly enhanced.
In this blog, we will explore how to automate data tasks with Python and Jupyter Notebooks. We’ll cover the basics of data automation, highlight Python libraries for automating data tasks, and demonstrate practical examples that showcase the ease of automating data workflows.
Table of Contents
Introduction to Data Automation
Benefits of Automating Data Tasks
Using Jupyter Notebooks for Data Automation
Popular Python Libraries for Data Automation
4.1. Pandas
4.2. NumPy
4.3. Openpyxl and xlrd (Excel Automation)
4.4. Selenium (Web Scraping Automation)
4.5. Requests and BeautifulSoup (API and Web Scraping)
4.6. sched and time (Scheduling Automation)
Example: Automating Data Processing with Pandas
Example: Automating Web Scraping with BeautifulSoup
Best Practices for Automating Data Tasks
Conclusion
1. Introduction to Data Automation
Data automation refers to the process of using software tools and scripts to automate the collection, processing, analysis, and reporting of data. This involves replacing manual tasks with automated workflows that can run on their own, typically without the need for human intervention. In Python, data automation tasks can be achieved through libraries and frameworks that provide functions for automating mundane processes.
Python's simplicity and versatility make it a go-to language for automating data workflows, ranging from simple data cleaning tasks to complex data processing operations. Jupyter Notebooks enhance this experience by offering an interactive environment where code, visualizations, and documentation can coexist, making it easy to experiment and document automation tasks.
2. Benefits of Automating Data Tasks
Time Savings: Automating repetitive tasks reduces the time spent on manual operations, allowing analysts and data scientists to focus on more important tasks.
Error Reduction: Automation minimizes human error, ensuring consistent results across multiple runs.
Scalability: Automated tasks can easily scale to handle larger datasets or more frequent data collection without requiring additional resources.
Increased Efficiency: Automation leads to faster processing and better utilization of computational resources.
Reproducibility: By automating processes, you can ensure that the same task can be reproduced with minimal effort, which is critical in research and production environments.
3. Using Jupyter Notebooks for Data Automation
Jupyter Notebooks are widely used in the data science and machine learning communities due to their ease of use, interactivity, and integration with Python. Jupyter provides an environment where you can write code, run it, visualize the output, and document your process, all in a single document.
When automating data tasks, Jupyter Notebooks can help you in the following ways:
Step-by-Step Execution: You can execute Python code step by step, enabling you to verify each part of the process before moving to the next step.
Inline Visualization: Jupyter allows you to display data visualizations directly within the notebook, making it easier to track the results of automation.
Documentation: Jupyter supports Markdown and LaTeX, so you can document your automation tasks clearly and concisely, making your process reproducible and understandable.
Here’s a simple example of how to use a Jupyter Notebook to automate a data processing task using Python:
Example:
# Importing required libraries
import pandas as pd
# Load dataset (Assume CSV file)
data = pd.read_csv('sales_data.csv')
# Automating data cleaning: Removing NaN values
data_cleaned = data.dropna()
# Automating data analysis: Calculating total sales
total_sales = data_cleaned['Sales'].sum()
# Outputting result
print(f"Total Sales: ${total_sales}")
In this example, Jupyter Notebooks helps to automate the process of loading a CSV file, cleaning the data by removing missing values, and calculating the total sales.
4. Popular Python Libraries for Data Automation
There are several Python libraries that are well-suited for automating various data-related tasks. Here are a few of the most popular ones:
4.1. Pandas
Pandas is the most widely used Python library for data manipulation and analysis. It provides easy-to-use data structures and functions to handle structured data. It simplifies tasks like cleaning, transforming, and aggregating data.
Example: Automating Data Cleaning with Pandasimport pandas as pd
# Load data
df = pd.read_csv('data.csv')
# Automate cleaning tasks (removing missing values)
df_cleaned = df.dropna()
# Automate grouping and summarizing data (e.g., total sales by region)
sales_by_region = df_cleaned.groupby('Region')['Sales'].sum()
# Save the cleaned and processed data
df_cleaned.to_csv('cleaned_data.csv')
With Pandas, we automate the cleaning and summarizing of the data, which can be especially useful when working with large datasets.
4.2. NumPy
NumPy is the fundamental library for numerical computing in Python. It provides support for large multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on them.
Example: Automating Numerical Calculations with NumPyimport numpy as np
# Automating array creation and mathematical operations
arr = np.array([1, 2, 3, 4, 5])
arr_squared = np.square(arr)
# Calculate mean and standard deviation
mean = np.mean(arr)
std_dev = np.std(arr)
# Output
print(f"Mean: {mean}, Standard Deviation: {std_dev}")
NumPy automates mathematical tasks on arrays and matrices, making it ideal for data processing and manipulation.
4.3. Openpyxl and xlrd (Excel Automation)
Openpyxl and xlrd are popular libraries for reading and writing Excel files. These libraries help automate tasks like data extraction, formatting, and reporting directly within Excel files.
Example: Automating Excel File Processing with Openpyxlfrom openpyxl import Workbook
# Create a new Excel workbook and add data
wb = Workbook()
ws = wb.active
ws['A1'] = 'Sales'
ws['A2'] = 5000
ws['A3'] = 7000
# Save the workbook
wb.save('sales_report.xlsx')
With Openpyxl, you can automate the process of creating, modifying, and saving Excel files for reports, which is a common task for many data professionals.
4.4. Selenium (Web Scraping Automation)
Selenium is a powerful library for automating web browsers. It is often used for scraping dynamic content from websites that require interaction (e.g., clicking buttons or filling forms).
Example: Automating Web Scraping with Seleniumfrom selenium import webdriver
# Open a web page
driver = webdriver.Chrome()
driver.get('https://example.com')
# Automate interaction (e.g., scraping data)
title = driver.title
print(f"Page Title: {title}")
# Close the browser
driver.quit()
Selenium allows you to automate the process of navigating and scraping web pages, making it valuable for automating data collection from websites.
4.5. Requests and BeautifulSoup (API and Web Scraping)
Requests and BeautifulSoup are other popular libraries for automating API calls and web scraping. Requests handle HTTP requests, while BeautifulSoup helps parse HTML and extract data.
Example: Automating API Calls and Data Extractionimport requests
from bs4 import BeautifulSoup
# Send a GET request to an API
response = requests.get('https://api.example.com/data')
# Parse JSON data from the API
data = response.json()
# Print data
print(data)
These libraries help automate the process of extracting data from APIs and web pages, which is common in many data automation workflows.
4.6. sched and time (Scheduling Automation)
For automating tasks on a schedule, you can use Python’s built-in sched and time libraries. They allow you to schedule tasks to run at specified times.
Example: Automating Scheduled Tasksimport time
import sched
# Initialize scheduler
scheduler = sched.scheduler(time.time, time.sleep)
# Define a task
def print_message():
print("Task Executed!")
# Schedule the task to run after 5 seconds
scheduler.enter(5, 1, print_message)
# Run the scheduled tasks
scheduler.run()
This example demonstrates how you can automate tasks to run at specific intervals using Python's scheduling libraries.
5. Example: Automating Data Processing with Pandas
Here’s a full example that automates a common data processing task using Pandas in a Jupyter Notebook.
import pandas as pd
# Load the dataset
data = pd.read_csv('sales_data.csv')
# Automate cleaning: Drop rows with missing values
clean_data = data.dropna()
# Automate transformation: Add a new column with discounted prices
clean_data['Discounted_Price'] = clean_data['Price'] * 0.9
# Automate summarization: Calculate total sales by product
total_sales_by_product = clean_data.groupby('Product')['Sales'].sum()
# Save the cleaned data
clean_data.to_csv('cleaned_sales_data.csv')
# Output the summary
print(total_sales_by_product)
6. Example: Automating Web Scraping with BeautifulSoup
Here’s how you can automate web scraping to collect data from a website.
import requests
from bs4 import BeautifulSoup
# Make a request to the website
url = 'https://example.com'
response = requests.get(url)
# Parse the HTML content
soup = BeautifulSoup(response.text, 'html.parser')
# Extract all headings (h1)
headings = soup.find_all('h1')
# Output the headings
for heading in headings:
print(heading.text)
7. Best Practices for Automating Data Tasks
Test your automation: Always test automated scripts with smaller datasets or in a controlled environment before running them at scale.
Error Handling: Implement proper error handling to ensure that your automation scripts can gracefully handle unexpected situations.
Logging: Use logging to track the progress and outcomes of automated tasks. This will help in debugging and auditing.
Modularize your code: Break down large automation tasks into smaller functions or modules to improve maintainability.
Document your process: Use Jupyter Notebooks or comments in your scripts to document your automation tasks for others to understand and reproduce.
8. Conclusion
Automating data tasks with Python and Jupyter Notebooks can save time, reduce errors, and help you streamline your data workflows. Whether you're working with data manipulation, web scraping, or scheduling tasks, Python provides a powerful toolkit to automate virtually every aspect of data processing. By using libraries such as Pandas, NumPy, Selenium, and BeautifulSoup, you can build efficient automation pipelines that handle data-related tasks with minimal manual effort. With Jupyter Notebooks, you can combine code, visualization, and documentation in one place, making it easier to experiment and share your work.
Start automating today to unlock more productive and efficient workflows.
Happy coding!