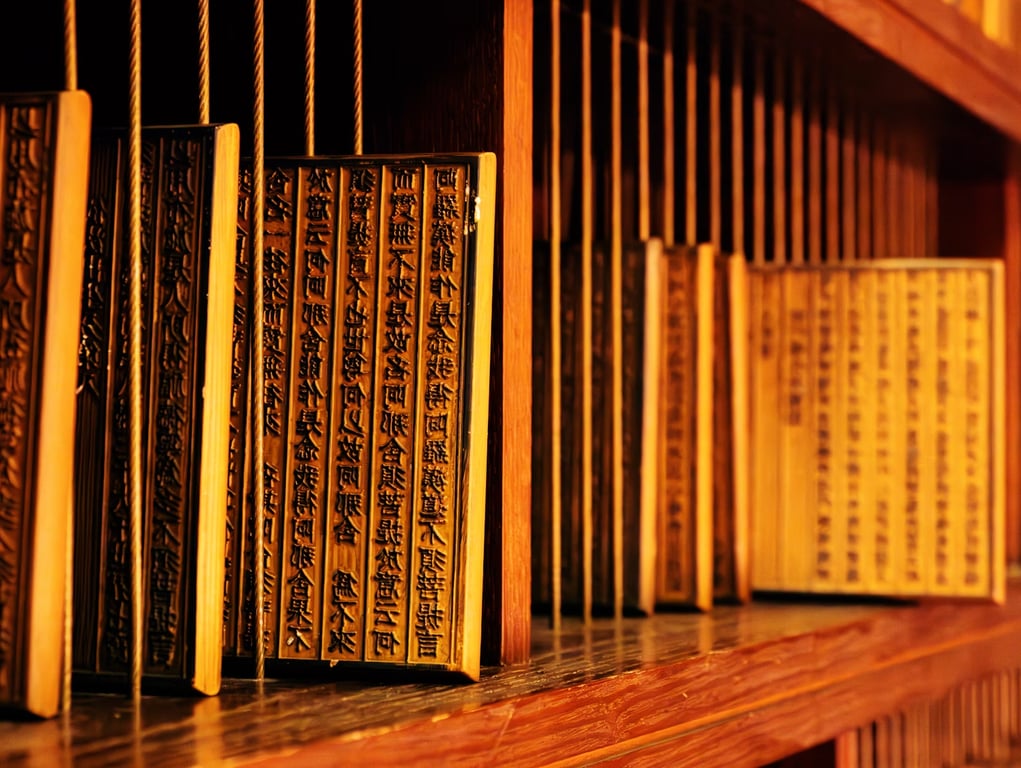
Photo by Declan Sun on Unsplash
Predictive analytics refers to the use of historical data, statistical algorithms, machine learning techniques, and artificial intelligence (AI) to predict future outcomes. In a world increasingly driven by data, businesses and individuals rely heavily on predictive analytics to make data-driven decisions. Python, with its rich ecosystem of libraries and frameworks, has become one of the most popular programming languages for predictive analytics.
In this blog post, we will explore how to use Python for predictive analytics. We’ll cover the basics of predictive analytics, the key steps in a predictive analytics project, popular Python libraries used for prediction, and walk through a complete example of building a predictive model.
Table of Contents
Introduction to Predictive Analytics
Key Steps in a Predictive Analytics Project
Popular Python Libraries for Predictive Analytics
3.1. Pandas
3.2. NumPy
3.3. Scikit-learn
3.4. XGBoost
3.5. Statsmodels
Preparing the Data for Predictive Analytics
Building and Training a Predictive Model
5.1. Linear Regression Example
Evaluating the Model’s Performance
Deploying the Model for Predictions
Best Practices in Predictive Analytics with Python
Conclusion
1. Introduction to Predictive Analytics
Predictive analytics is the process of analyzing historical data and making predictions about future events or trends. It utilizes a combination of statistical techniques, machine learning algorithms, and AI to build models that can identify patterns and forecast outcomes. Predictive analytics is widely used in various industries, including finance, healthcare, marketing, sales, and more.
In the context of Python, predictive analytics can be easily implemented using various libraries that provide the tools necessary for building machine learning models, performing statistical analysis, and visualizing results.
2. Key Steps in a Predictive Analytics Project
A typical predictive analytics project can be broken down into several key steps:
2.1. Define the Problem
The first step is to clearly define the problem that you are trying to solve. This could be predicting sales, identifying customer churn, forecasting stock prices, or anything else that requires a future prediction.
2.2. Collect Data
Next, you need to collect the data that will be used to train the model. Data can come from various sources, including databases, CSV files, APIs, web scraping, or other data repositories.
2.3. Prepare and Clean the Data
Data preparation involves cleaning the data (e.g., handling missing values, encoding categorical variables, scaling features) and transforming it into a format suitable for training the model.
2.4. Train the Model
Once the data is prepared, you can use machine learning algorithms to train a predictive model. This involves selecting the appropriate algorithm and adjusting the model parameters.
2.5. Evaluate the Model
After training, you must evaluate the model's performance using various metrics such as accuracy, precision, recall, F1-score, etc., depending on the type of prediction you are making (e.g., classification or regression).
2.6. Deploy the Model
Finally, once the model is trained and evaluated, it can be deployed to make real-time predictions on new data.
3. Popular Python Libraries for Predictive Analytics
Several Python libraries are commonly used for building predictive analytics models. Below are some of the most popular ones:
3.1. Pandas
Pandas is a powerful library for data manipulation and analysis. It provides data structures like DataFrames and Series, which are essential for handling and preparing data for predictive analytics.
3.2. NumPy
NumPy is a core library for numerical computing in Python. It provides support for arrays and matrices, along with a variety of mathematical functions that are useful in data analysis and building predictive models.
3.3. Scikit-learn
Scikit-learn is one of the most widely used libraries for machine learning in Python. It provides simple and efficient tools for data mining and data analysis, including tools for classification, regression, clustering, and dimensionality reduction.
3.4. XGBoost
XGBoost is a high-performance implementation of gradient boosting algorithms. It’s widely used in machine learning competitions due to its ability to handle large datasets and produce highly accurate models.
3.5. Statsmodels
Statsmodels is a library for statistical modeling in Python. It includes tools for performing regression analysis, hypothesis testing, time-series analysis, and other statistical methods.
4. Preparing the Data for Predictive Analytics
Before you can build a predictive model, it’s essential to prepare your data. This process involves cleaning and transforming the raw data into a format that can be fed into machine learning algorithms. Here are some common steps in data preparation:
4.1. Handle Missing Data
Missing values in a dataset can be dealt with by removing rows or columns with missing data, or imputing values using techniques like mean or median imputation.
4.2. Encode Categorical Variables
Machine learning algorithms often require numeric data, so categorical variables need to be encoded. One-hot encoding is a common technique used to convert categorical data into a format suitable for machine learning.
4.3. Scale the Data
Feature scaling is important to ensure that features are on the same scale, especially when using distance-based algorithms like k-Nearest Neighbors (k-NN) or Support Vector Machines (SVM).
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
scaled_data = scaler.fit_transform(data)
5. Building and Training a Predictive Model
Once the data is ready, you can build and train a predictive model. Let’s take a look at a simple example using Linear Regression to predict house prices based on various features like size, location, and number of rooms.
5.1. Linear Regression Example
# Import necessary libraries
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
# Load the dataset
data = pd.read_csv('housing_data.csv')
# Select features and target
X = data[['Size', 'Bedrooms', 'Location']]
y = data['Price']
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train the model
model = LinearRegression()
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
mse = mean_squared_error(y_test, y_pred)
print(f"Mean Squared Error: {mse}")
In this example, we train a linear regression model to predict house prices based on features like size, number of bedrooms, and location. We evaluate the model using the Mean Squared Error (MSE) metric.
6. Evaluating the Model’s Performance
Once the model has been trained, it’s crucial to evaluate its performance. Common evaluation metrics include:
Accuracy: The proportion of correctly predicted instances (for classification tasks).
Mean Squared Error (MSE): The average squared difference between predicted and actual values (for regression tasks).
Confusion Matrix: A table that helps evaluate the performance of a classification model by showing the true positives, true negatives, false positives, and false negatives.
Example: Evaluation Metrics for Classification
from sklearn.metrics import accuracy_score, confusion_matrix
# Assume y_test and y_pred are the true and predicted labels
accuracy = accuracy_score(y_test, y_pred)
conf_matrix = confusion_matrix(y_test, y_pred)
print(f"Accuracy: {accuracy}")
print(f"Confusion Matrix: \n{conf_matrix}")
Example: Evaluation Metrics for Regression
from sklearn.metrics import mean_absolute_error, mean_squared_error
mae = mean_absolute_error(y_test, y_pred)
mse = mean_squared_error(y_test, y_pred)
print(f"Mean Absolute Error: {mae}")
print(f"Mean Squared Error: {mse}")
7. Deploying the Model for Predictions
After training and evaluating the model, the next step is to deploy it. This can involve integrating the model into a production system, using it to make real-time predictions, or creating an API to serve predictions.
For deployment, you can use Python web frameworks like Flask or Django, or you can use cloud platforms like AWS Lambda, Google Cloud Functions, or Azure Functions to deploy your model.
Example: Simple Flask API for Model Prediction
from flask import Flask, request, jsonify
import pickle
# Load the trained model
model = pickle.load(open('model.pkl', 'rb'))
app = Flask(__name__)
@app.route('/predict', methods=['POST'])
def predict():
data = request.get_json() # Get data from POST request
prediction = model.predict([data['features']])
return jsonify({'prediction': prediction[0]})
if __name__ == '__main__':
app.run(debug=True)
This simple Flask API loads a trained model and uses it to make predictions based on incoming data.
8. Best Practices in Predictive Analytics with Python
Data Preprocessing: Always spend sufficient time on data cleaning and preparation. A well-prepared dataset leads to better model performance.
Feature Engineering: Create new features from existing ones to improve the model’s predictive power.
Model Tuning: Tune hyperparameters to optimize model performance.
Cross-Validation: Use techniques like k-fold cross-validation to avoid overfitting and ensure generalization.
Model Monitoring: After deployment, monitor the model’s performance and retrain it periodically with new data.
9. Conclusion
Python provides a robust environment for building predictive models, with libraries such as Scikit-learn, Pandas, and XGBoost making it easier to implement machine learning and statistical techniques. Predictive analytics is a powerful tool that can provide valuable insights and help organizations make data-driven decisions. By following the key steps outlined in this blog post, you can start building your own predictive models and leveraging them to forecast future trends.
Happy coding!