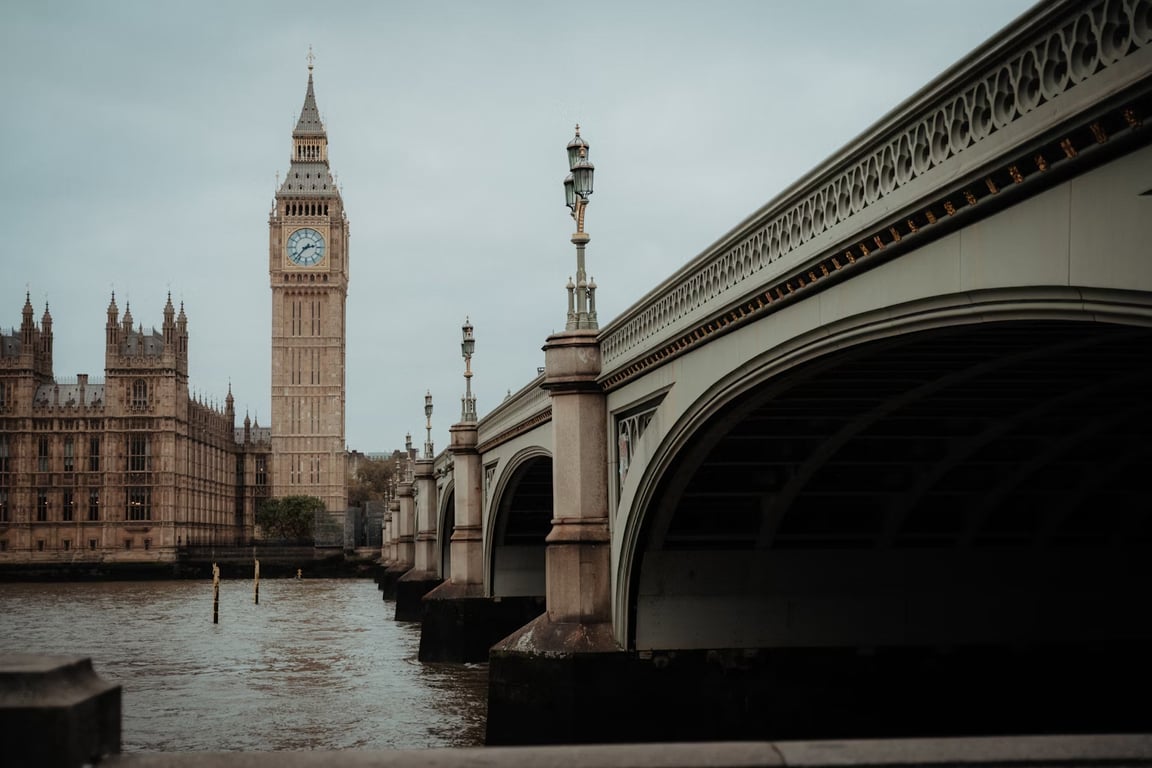
Photo by Luise and Nic on Unsplash
Machine learning (ML) has revolutionized various industries by enabling systems to learn from data and make predictions or decisions without explicit programming. While many popular machine learning frameworks like scikit-learn, TensorFlow, and PyTorch offer pre-built algorithms, understanding how to implement these algorithms from scratch can deepen your understanding of the concepts and improve your ability to customize them for specific needs.
In this blog post, we’ll walk through how to implement your own machine learning algorithms in Python. By doing so, you'll learn the underlying mechanics of machine learning algorithms, which can empower you to create efficient and customized solutions. We’ll cover the steps involved, use simple Python libraries, and walk through practical examples for a clear understanding.
Why Implement Machine Learning Algorithms from Scratch?
Before diving into the code, let's understand why implementing algorithms from scratch can be valuable:
Deep Understanding: It gives you a fundamental understanding of how machine learning algorithms work under the hood.
Customization: Building algorithms yourself allows you to modify and tailor them for your specific dataset or problem.
Performance Optimization: Understanding the algorithm’s intricacies allows for performance optimization, such as improving speed or accuracy.
Educational Value: If you're learning machine learning, implementing algorithms yourself strengthens the core concepts and enhances your learning experience.
Now that we understand why it's beneficial, let’s break down the process of implementing machine learning algorithms.
Steps for Implementing a Machine Learning Algorithm
Choose a Simple Algorithm: Start with simple algorithms such as linear regression, k-nearest neighbors, or decision trees.
Understand the Math Behind It: Before coding, understand the mathematical foundation of the algorithm. This step helps ensure you correctly implement the algorithm.
Prepare Your Dataset: Clean and preprocess the data. Machine learning algorithms rely on high-quality, well-prepared data.
Write the Algorithm: Write the code for the algorithm step by step, ensuring that each operation adheres to the algorithm's logic.
Train and Test the Algorithm: Train your model using a dataset, then test the model’s performance to see how well it generalizes to unseen data.
Optimize and Tune the Algorithm: Fine-tune parameters, improve efficiency, or make adjustments to increase the algorithm's accuracy.
Now, let’s go through a few examples to help you understand how to implement machine learning algorithms from scratch in Python.
Implementing Linear Regression from Scratch
Linear regression is one of the simplest and most widely used machine learning algorithms for predicting continuous values based on linear relationships between the input features and the target variable.
What is Linear Regression?
Linear regression assumes that there is a linear relationship between the input features (X) and the target variable (y). The equation of a line is represented as:
y=w0+w1x1+w2x2+⋯+wnxn
Where:
yyy is the predicted output.
x1,x2,…,xnx_1, x_2, \dots, x_nx1,x2,…,xn are the input features.
w0,w1,…,wnw_0, w_1, \dots, w_nw0,w1,…,wn are the weights (coefficients) that the algorithm will learn.
Steps to Implement Linear Regression
Initialize weights: Randomly initialize the weights (parameters) of the model.
Calculate predictions: Use the formula to calculate predictions.
Compute the loss function: The loss function measures the difference between the predicted values and the actual values.
Gradient Descent: Update the weights by moving in the direction that reduces the loss (using the gradient of the loss function).
Repeat: Repeat the process until the model converges or reaches a specified number of iterations.
Here’s a simple implementation:
import numpy as np
class LinearRegression:
def __init__(self, learning_rate=0.01, n_iterations=1000):
self.learning_rate = learning_rate
self.n_iterations = n_iterations
self.weights = None
def fit(self, X, y):
n_samples, n_features = X.shape
self.weights = np.zeros(n_features)
for _ in range(self.n_iterations):
y_pred = self.predict(X)
loss = y_pred - y
# Gradient descent update rule
for i in range(n_features):
self.weights[i] -= self.learning_rate * (2 / n_samples) * np.dot(loss, X[:, i])
def predict(self, X):
return np.dot(X, self.weights)
# Example usage
X_train = np.array([[1, 2], [2, 3], [3, 4], [4, 5]]) # Example input features
y_train = np.array([5, 7, 9, 11]) # Example target variable
model = LinearRegression()
model.fit(X_train, y_train)
predictions = model.predict(X_train)
print(predictions)
Explanation of the Code:
Initialization: The model starts by initializing the weights to zeros.
Loss and Gradient: The difference between the predicted values and the true values (loss) is used to adjust the weights using gradient descent.
Prediction: After training, we use the
predict()
function to make predictions on new data.
Implementing k-Nearest Neighbors (k-NN) from Scratch
k-NN is a simple and effective algorithm used for classification and regression tasks. It works by finding the k-nearest data points in the feature space and using those points to predict the target variable.
What is k-Nearest Neighbors?
The k-NN algorithm predicts the class or value of a data point based on the classes or values of its k-nearest neighbors in the feature space. The Euclidean distance is typically used to measure the proximity between data points.
Steps to Implement k-NN
Calculate distance: Compute the distance between the query point and all other data points in the dataset.
Sort distances: Sort the data points by distance.
Select k nearest neighbors: Choose the k nearest points.
Predict: For classification, return the majority class; for regression, return the mean value of the k nearest points.
Here’s an implementation of k-NN for classification:
import numpy as np
from collections import Counter
class KNearestNeighbors:
def __init__(self, k=3):
self.k = k
def fit(self, X_train, y_train):
self.X_train = X_train
self.y_train = y_train
def predict(self, X_test):
predictions = [self._predict(x) for x in X_test]
return np.array(predictions)
def _predict(self, x):
# Compute the Euclidean distance between x and all training examples
distances = [np.linalg.norm(x - x_train) for x_train in self.X_train]
# Get the indices of the k smallest distances
k_indices = np.argsort(distances)[:self.k]
# Get the labels of the k nearest neighbors
k_nearest_labels = [self.y_train[i] for i in k_indices]
# Return the most common class label
most_common = Counter(k_nearest_labels).most_common(1)
return most_common[0][0]
# Example usage
X_train = np.array([[1, 2], [2, 3], [3, 4], [5, 5]]) # Input features
y_train = np.array([0, 1, 1, 0]) # Labels
X_test = np.array([[3, 3]]) # Test data
model = KNearestNeighbors(k=2)
model.fit(X_train, y_train)
prediction = model.predict(X_test)
print(prediction)
Explanation of the Code:
Euclidean Distance: The
_predict()
method computes the distance between the query point and all training samples.Majority Voting: For classification, the most common label among the k-nearest neighbors is selected.
Challenges and Advanced Concepts in Implementing Machine Learning Algorithms
Feature Scaling: Many machine learning algorithms require feature scaling to work effectively. For instance, algorithms like k-NN and linear regression can be sensitive to the scale of features, and thus, normalization or standardization may be necessary.
Overfitting and Underfitting: When implementing algorithms from scratch, it's important to understand and handle overfitting (model is too complex) and underfitting (model is too simple). Regularization techniques like L1 and L2 regularization can help mitigate these issues.
Optimization: While implementing machine learning algorithms from scratch, you'll need to tune hyperparameters like the learning rate, number of iterations, or the value of k in k-NN. Understanding optimization techniques such as gradient descent can help improve the performance of your model.
Vectorization: For larger datasets, Python loops can be slow. Optimizing the implementation by using vectorized operations (with libraries like NumPy) can significantly speed up your code.
Model Evaluation: It's crucial to evaluate your models using metrics such as accuracy, precision, recall, and F1-score (for classification) or mean squared error (for regression). This step is essential to assess whether the algorithm is working effectively.
Conclusion
In this blog post, we demonstrated how to implement two classic machine learning algorithms—linear regression and k-nearest neighbors—from scratch in Python. Implementing these algorithms yourself provides a solid understanding of their inner workings and equips you with the knowledge to customize and optimize them as per your needs.
Remember that the journey of building machine learning algorithms from scratch is an ongoing learning experience. As you implement more complex algorithms and techniques, you'll continue to deepen your understanding of machine learning and become more proficient at solving real-world problems with Python.
By mastering the basics and understanding the math and logic behind machine learning algorithms, you’ll be better prepared to tackle challenges and build your own models for various applications.
Happy coding!