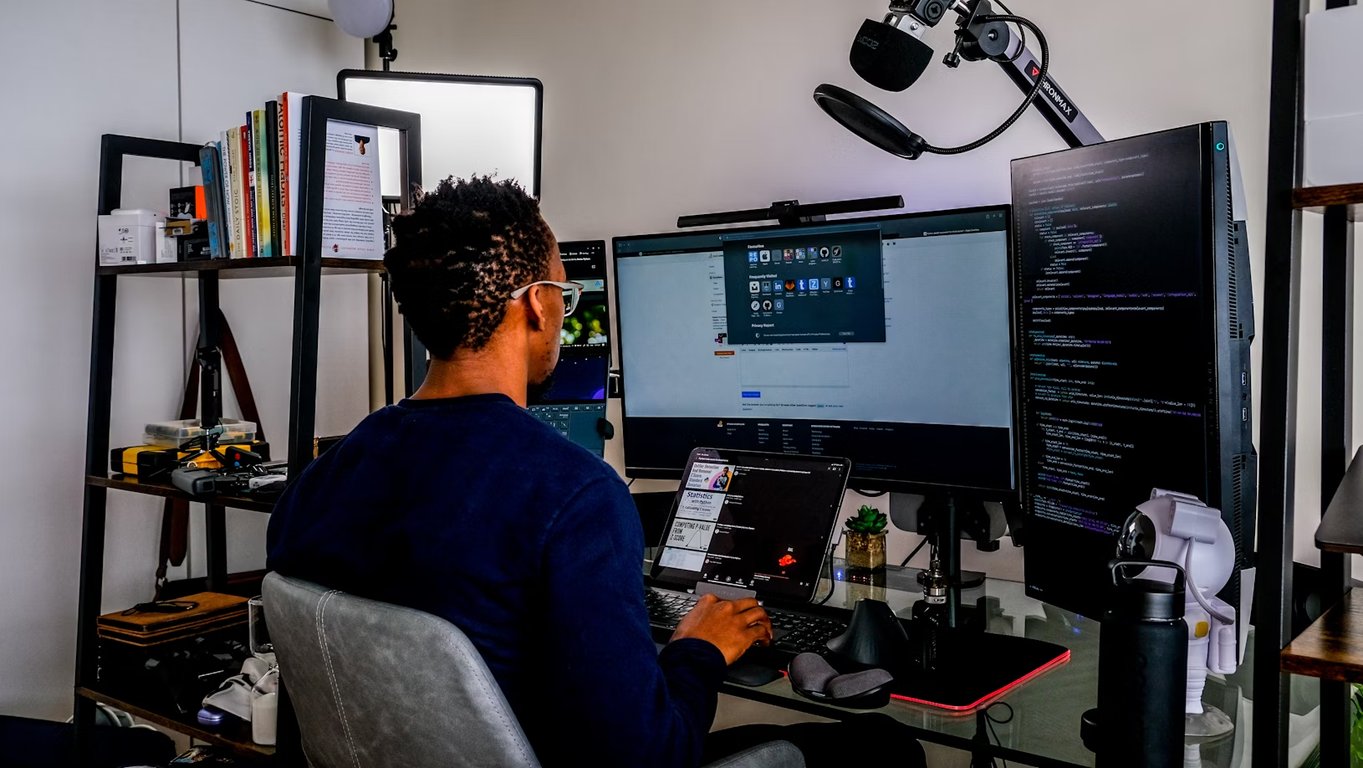
Photo by Boitumelo on Unsplash
Deep learning has revolutionized fields like image recognition, natural language processing, and game-playing AI. At the core of deep learning are artificial neural networks, and TensorFlow and Keras are two of the most widely used libraries for building these models. This blog will introduce you to the basics of deep learning and provide practical examples using TensorFlow and Keras in Python.
Table of Contents
What is Deep Learning?
Introduction to TensorFlow and Keras
Setting Up Your Environment
Basic Components of a Neural Network
Building Your First Deep Learning Model with Keras
5.1. Dataset Preparation
5.2. Creating a Neural Network
5.3. Compiling the Model
5.4. Training and Evaluating the Model
Understanding Model Performance
Saving and Loading Models
Common Use Cases of Deep Learning
Tips for Beginners
Conclusion
1. What is Deep Learning?
Deep learning is a subset of machine learning that uses artificial neural networks to learn patterns from data. These networks, inspired by the human brain, consist of layers of interconnected nodes (neurons) that process information.
Key Features of Deep Learning
Feature Extraction: Automatically learns relevant features from raw data.
Scalability: Handles large datasets effectively.
Versatility: Applicable to images, text, audio, and more.
2. Introduction to TensorFlow and Keras
TensorFlow
TensorFlow is an open-source deep learning framework developed by Google. It provides tools for building and deploying machine learning models.
Keras
Keras is a high-level API built on top of TensorFlow. It simplifies the process of designing, training, and deploying deep learning models.
3. Setting Up Your Environment
To start with TensorFlow and Keras, install the libraries:
pip install tensorflow
Verify the installation:
import tensorflow as tf
print(tf.__version__)
4. Basic Components of a Neural Network
A neural network consists of:
Input Layer: Takes the input data.
Hidden Layers: Perform computations and extract features.
Output Layer: Produces predictions.
Example
A neural network for classifying handwritten digits (e.g., MNIST dataset) might look like:
Input Layer: 784 neurons (28x28 pixel images)
Hidden Layer: 128 neurons
Output Layer: 10 neurons (digits 0-9)
5. Building Your First Deep Learning Model with Keras
5.1. Dataset Preparation
Keras provides built-in datasets like MNIST. Let’s use it for this example:
from tensorflow.keras.datasets import mnist
# Load dataset
(X_train, y_train), (X_test, y_test) = mnist.load_data()
# Normalize the data
X_train = X_train / 255.0
X_test = X_test / 255.0
5.2. Creating a Neural Network
Define a simple feedforward neural network using the Sequential
API:
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Flatten, Dense
model = Sequential([
Flatten(input_shape=(28, 28)), # Flatten the 2D images to 1D
Dense(128, activation='relu'), # Hidden layer with 128 neurons
Dense(10, activation='softmax') # Output layer with 10 neurons
])
5.3. Compiling the Model
Compile the model by specifying:
Loss Function: Measures the difference between predictions and true values.
Optimizer: Updates weights to minimize the loss.
Metrics: Evaluates model performance.
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
5.4. Training and Evaluating the Model
Train the model using the training data and evaluate it on the test set:
model.fit(X_train, y_train, epochs=5) # Train for 5 epochs
test_loss, test_accuracy = model.evaluate(X_test, y_test)
print(f"Test Accuracy: {test_accuracy:.2f}")
6. Understanding Model Performance
Visualize the model’s predictions:
import numpy as np
predictions = model.predict(X_test)
predicted_labels = np.argmax(predictions, axis=1)
# Compare predictions with actual labels
print(f"True Label: {y_test[0]}, Predicted Label: {predicted_labels[0]}")
7. Saving and Loading Models
Save the trained model for future use:
model.save('mnist_model.h5')
Load the saved model:
from tensorflow.keras.models import load_model
loaded_model = load_model('mnist_model.h5')
8. Common Use Cases of Deep Learning
Image Recognition: Classifying objects in images.
Natural Language Processing: Language translation, sentiment analysis.
Speech Recognition: Transcribing audio to text.
Recommendation Systems: Suggesting products or content.
Autonomous Vehicles: Detecting objects and navigating.
9. Tips for Beginners
Start Small: Begin with simple models and datasets.
Experiment: Try different architectures and hyperparameters.
Use Pre-Trained Models: Leverage models like ResNet or BERT for complex tasks.
Understand the Basics: Focus on concepts like backpropagation and gradient descent.
10. Conclusion
Deep learning is a powerful tool for solving complex problems across various domains. With TensorFlow and Keras, building and training neural networks becomes accessible even to beginners. Start with the basics, experiment with different architectures, and gradually explore advanced techniques to unlock the full potential of deep learning.
Happy coding!