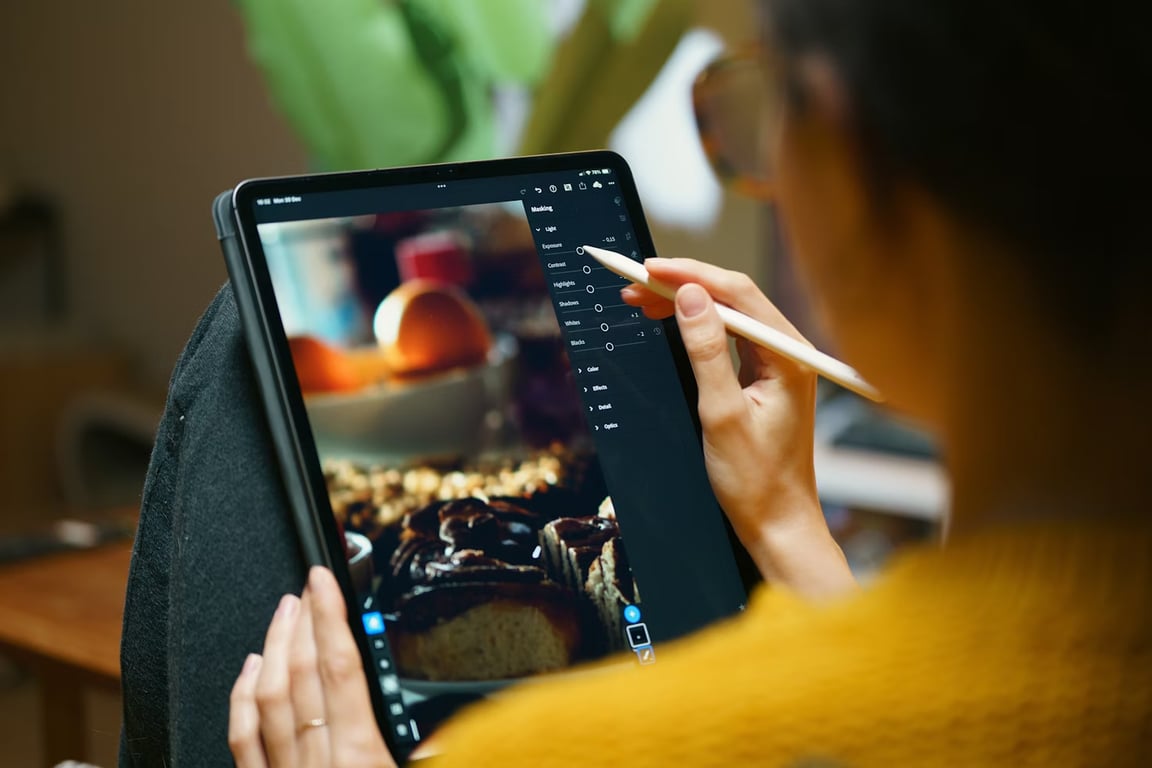
Photo by Jakub Żerdzicki on Unsplash
Sentiment analysis is one of the most popular natural language processing (NLP) tasks. It involves determining the emotional tone behind a piece of text, which can help in understanding opinions, attitudes, and emotions. From analyzing customer reviews to monitoring social media sentiment, this technique has a wide range of applications.
In this blog, we’ll explore how to perform sentiment analysis using Python and various NLP libraries. By the end, you’ll have a clear understanding of the key concepts, tools, and steps involved in implementing sentiment analysis.
Table of Contents
What is Sentiment Analysis?
Applications of Sentiment Analysis
How Sentiment Analysis Works
Setting Up Your Environment
Step-by-Step Guide to Sentiment Analysis
5.1. Data Collection
5.2. Text Preprocessing
5.3. Tokenization
5.4. Feature Extraction
5.5. Model Training and Testing
Using Pre-Trained Models for Sentiment Analysis
Evaluating the Model
Advanced Techniques in Sentiment Analysis
Challenges in Sentiment Analysis
Conclusion
1. What is Sentiment Analysis?
Sentiment analysis, also known as opinion mining, is the process of using NLP and text analysis to identify and extract subjective information from text. It typically classifies text into predefined sentiment categories such as positive, negative, or neutral.
For example:
Text: "The movie was fantastic!"
Sentiment: Positive
Text: "The service was terrible and disappointing."
Sentiment: Negative
2. Applications of Sentiment Analysis
Sentiment analysis is used in various fields, including:
Customer Feedback: Analyzing reviews, ratings, and surveys to understand customer satisfaction.
Social Media Monitoring: Tracking brand perception and public sentiment on platforms like Twitter.
Market Research: Studying consumer opinions to identify trends and preferences.
Political Analysis: Assessing public opinion on political issues or events.
Healthcare: Identifying mental health patterns through social media posts or surveys.
3. How Sentiment Analysis Works
Sentiment analysis typically involves these steps:
Data Collection: Gathering textual data from reviews, tweets, or other sources.
Text Preprocessing: Cleaning and preparing the text for analysis.
Tokenization: Breaking the text into smaller units, such as words or phrases.
Feature Extraction: Converting text into numerical representations using techniques like TF-IDF or word embeddings.
Classification: Applying a machine learning or deep learning model to classify the sentiment.
4. Setting Up Your Environment
To perform sentiment analysis in Python, you need the following libraries:
NLTK: Natural Language Toolkit for text preprocessing.
TextBlob: A simple library for sentiment analysis.
VADER: A lexicon and rule-based sentiment analysis tool.
scikit-learn: For building and evaluating machine learning models.
Install the required libraries using pip:
pip install nltk textblob vaderSentiment scikit-learn
5. Step-by-Step Guide to Sentiment Analysis
5.1. Data Collection
For this example, let’s use sample customer reviews:
reviews = [
"I love this product! It's amazing.",
"The experience was horrible. I will not buy again.",
"It's okay, nothing special.",
"Absolutely fantastic! Highly recommended.",
"Terrible quality, very disappointed."
]
You can also load data from files or APIs.
5.2. Text Preprocessing
Text preprocessing involves cleaning the text by removing unnecessary elements like punctuation, stopwords, and special characters.
import re
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
import nltk
nltk.download('stopwords')
nltk.download('punkt')
def preprocess_text(text):
# Convert to lowercase
text = text.lower()
# Remove punctuation and special characters
text = re.sub(r'[^\w\s]', '', text)
# Tokenize
tokens = word_tokenize(text)
# Remove stopwords
tokens = [word for word in tokens if word not in stopwords.words('english')]
return ' '.join(tokens)
cleaned_reviews = [preprocess_text(review) for review in reviews]
print(cleaned_reviews)
5.3. Tokenization
Tokenization splits the text into individual words, phrases, or sentences. This step helps in understanding the structure of the text.
5.4. Feature Extraction
Convert text into numerical features using methods like Bag-of-Words or TF-IDF.
from sklearn.feature_extraction.text import CountVectorizer
vectorizer = CountVectorizer()
X = vectorizer.fit_transform(cleaned_reviews)
print(vectorizer.get_feature_names_out())
5.5. Model Training and Testing
Train a machine learning model like Naive Bayes for sentiment classification.
from sklearn.model_selection import train_test_split
from sklearn.naive_bayes import MultinomialNB
from sklearn.metrics import accuracy_score
# Sample labels for demonstration (1: Positive, 0: Negative)
labels = [1, 0, 0, 1, 0]
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, labels, test_size=0.2, random_state=42)
# Train model
model = MultinomialNB()
model.fit(X_train, y_train)
# Predict
predictions = model.predict(X_test)
print(f"Accuracy: {accuracy_score(y_test, predictions)}")
6. Using Pre-Trained Models for Sentiment Analysis
Libraries like TextBlob and VADER provide pre-trained sentiment analysis models.
Using TextBlob
from textblob import TextBlob
for review in reviews:
sentiment = TextBlob(review).sentiment.polarity
print(f"Review: {review} | Sentiment Score: {sentiment}")
Using VADER
from vaderSentiment.vaderSentiment import SentimentIntensityAnalyzer
analyzer = SentimentIntensityAnalyzer()
for review in reviews:
sentiment = analyzer.polarity_scores(review)
print(f"Review: {review} | Sentiment: {sentiment}")
7. Evaluating the Model
Evaluate the performance of your sentiment analysis model using metrics like:
Accuracy
Precision
Recall
F1-Score
8. Advanced Techniques in Sentiment Analysis
Deep Learning: Use LSTMs, GRUs, or transformers like BERT for more complex sentiment analysis.
Word Embeddings: Leverage word embeddings like Word2Vec or GloVe for feature representation.
Fine-Tuning: Fine-tune pre-trained models like BERT or GPT for sentiment classification tasks.
9. Challenges in Sentiment Analysis
Sarcasm Detection: Identifying sarcastic tones can be difficult.
Ambiguity: Text with mixed sentiments can be hard to classify.
Domain-Specific Vocabulary: Sentiments may vary across different industries.
10. Conclusion
Sentiment analysis is a powerful tool for extracting emotions and opinions from text. Using Python and NLP libraries, you can build efficient models to classify sentiments in various datasets. Start with basic approaches like TextBlob and VADER, and gradually explore advanced techniques like deep learning and transformer-based models.
Experiment with real-world datasets, refine your models, and harness the power of sentiment analysis to drive insights from text data.
Happy coding!