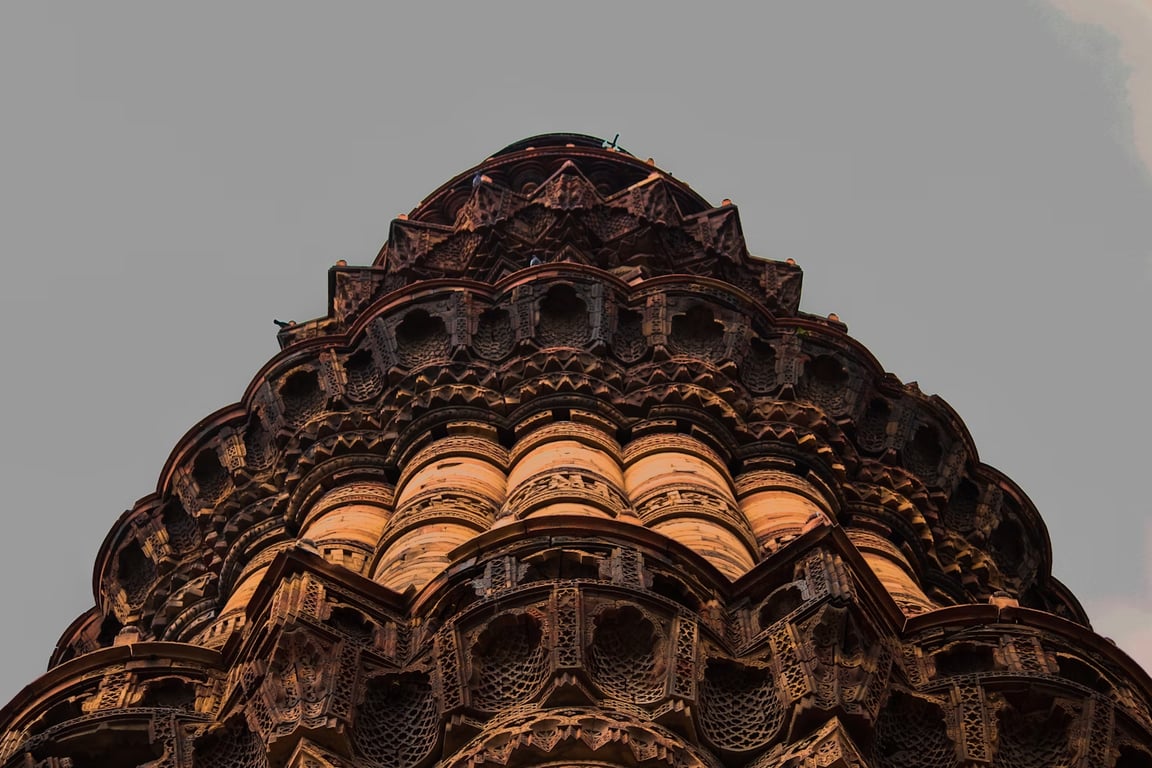
Python has become one of the most popular programming languages for data analysis, and at the heart of this popularity is the pandas library. Pandas provides powerful, flexible, and easy-to-use data structures that are essential for handling structured data, such as CSV files, SQL databases, and Excel spreadsheets. Whether you're working with small or large datasets, pandas helps you perform a wide range of data manipulation, cleaning, and analysis tasks efficiently.
In this guide, we will explore pandas, explain its core components, and show how you can use it to perform data analysis. By the end of this blog, you'll be comfortable with the basics of pandas and ready to dive into more advanced techniques.
1. What is pandas?
pandas is an open-source Python library that provides data structures and functions for efficiently manipulating large datasets. Its name is derived from the term “panel data,” which refers to multi-dimensional data sets. pandas allows you to work with two primary data structures:
Series: A one-dimensional labeled array (similar to a list or array in Python).
DataFrame: A two-dimensional labeled data structure (similar to a table or spreadsheet).
pandas is built on top of NumPy, which means it leverages NumPy’s array capabilities for performance. It also integrates well with other libraries such as matplotlib for visualization and scikit-learn for machine learning.
2. Setting Up pandas
Before we begin using pandas, you need to install it. You can install pandas via pip:
pip install pandas
Once pandas is installed, you can import it into your Python script:
import pandas as pd
By convention, pandas is imported as pd
, which makes the code cleaner and easier to read.
3. Understanding pandas Data Structures
3.1. Series
A Series is essentially a one-dimensional array with labels (also called index). You can think of it as a list, but with an index attached to each element. The Series object is the foundation of pandas DataFrames.
Example of creating a Series:import pandas as pd
# Create a Series
data = [10, 20, 30, 40, 50]
index = ['a', 'b', 'c', 'd', 'e']
series = pd.Series(data, index=index)
print(series)
Output:
a 10
b 20
c 30
d 40
e 50
dtype: int64
In this example, the index labels are 'a', 'b', 'c', 'd', and 'e', while the data values are 10, 20, 30, 40, and 50.
3.2. DataFrame
A DataFrame is a two-dimensional table, similar to a spreadsheet or SQL table, with rows and columns. Each column can be a different data type (integer, float, string, etc.). A DataFrame is built from Series, where each Series represents a column.
Example of creating a DataFrame:import pandas as pd
# Create a DataFrame
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
print(df)
Output:
Name Age City
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
Each row represents an individual record, and each column represents a specific attribute (Name, Age, City).
4. Basic Operations with pandas
4.1. Reading Data into pandas
You can load data from various file formats into pandas using functions like read_csv()
, read_excel()
, and read_sql()
. The most common method is reading a CSV file.
import pandas as pd
# Load a CSV file into a DataFrame
df = pd.read_csv('data.csv')
# Display the first few rows
print(df.head())
The head()
function shows the first five rows of the DataFrame by default. You can also use df.tail()
to view the last few rows.
4.2. Exploring DataFrames
Once you've loaded the data, there are several ways to explore it:
Checking the shape of the DataFrame:
print(df.shape) # (rows, columns)
Viewing data types:
print(df.dtypes)
ummary statistics:
print(df.describe())
Checking for missing values:
print(df.isnull().sum())
4.3. Data Selection and Indexing
You can access specific rows and columns of a DataFrame using various methods:
Selecting a single column:
print(df['Age'])
Selecting multiple columns:
print(df[['Name', 'City']])
Selecting rows by index:
print(df.iloc[0]) # First row
Selecting rows based on conditions:
print(df[df['Age'] > 30])
5. Data Cleaning with pandas
5.1. Handling Missing Data
Dealing with missing data is a common task in data analysis. pandas provides functions to handle missing values.
Identifying missing data:
print(df.isnull())
Dropping rows with missing values:
df = df.dropna()
Filling missing values:
df = df.fillna(0) # Fill missing values with 0
5.2. Removing Duplicates
You can remove duplicate rows from your DataFrame using the drop_duplicates()
method.
df = df.drop_duplicates()
5.3. Changing Data Types
To change the data type of a column, you can use the astype()
method.
df['Age'] = df['Age'].astype(float)
6. Data Manipulation with pandas
6.1. Sorting and Filtering Data
You can sort and filter data easily with pandas:
Sorting data:
df = df.sort_values(by='Age', ascending=False)
Filtering data:
filtered_df = df[df['City'] == 'New York']
6.2. Grouping Data
You can group data by one or more columns and perform aggregate operations like sum, mean, or count.
grouped = df.groupby('City').agg({'Age': 'mean'})
print(grouped)
6.3. Merging and Joining DataFrames
You can merge or join multiple DataFrames based on common columns or indexes.
df1 = pd.DataFrame({'ID': [1, 2, 3], 'Name': ['Alice', 'Bob', 'Charlie']})
df2 = pd.DataFrame({'ID': [2, 3, 4], 'Age': [30, 35, 40]})
merged_df = pd.merge(df1, df2, on='ID')
print(merged_df)
7. Data Analysis and Visualization
7.1. Summary Statistics
Pandas makes it easy to compute summary statistics:
Mean, median, and standard deviation:
print(df['Age'].mean()) print(df['Age'].median()) print(df['Age'].std())
Correlation:
print(df.corr())
7.2. Data Visualization with pandas
Pandas also has built-in support for basic plotting with the plot()
function, which relies on matplotlib.
import matplotlib.pyplot as plt
df['Age'].plot(kind='hist')
plt.show()
8. Advanced pandas Features
Pandas also includes powerful features like:
Pivot Tables: Create pivot tables to summarize data.
Time Series Analysis: Handle time-based data using the
pd.to_datetime()
function.Categorical Data: Optimize memory usage by using categorical types for repeated strings.
9. Conclusion
In this beginner's guide to pandas, we’ve covered the fundamentals of data analysis with pandas, from reading and exploring data to cleaning, manipulating, and analyzing it. Pandas is a powerful tool that provides intuitive data structures and functions that make data analysis more accessible and efficient.
By mastering pandas, you can tackle a wide range of data analysis tasks with ease, enabling you to unlock valuable insights from your data. As you become more comfortable with pandas, you’ll discover more advanced features and techniques that will help you take your data analysis skills to the next level.
Happy coding!