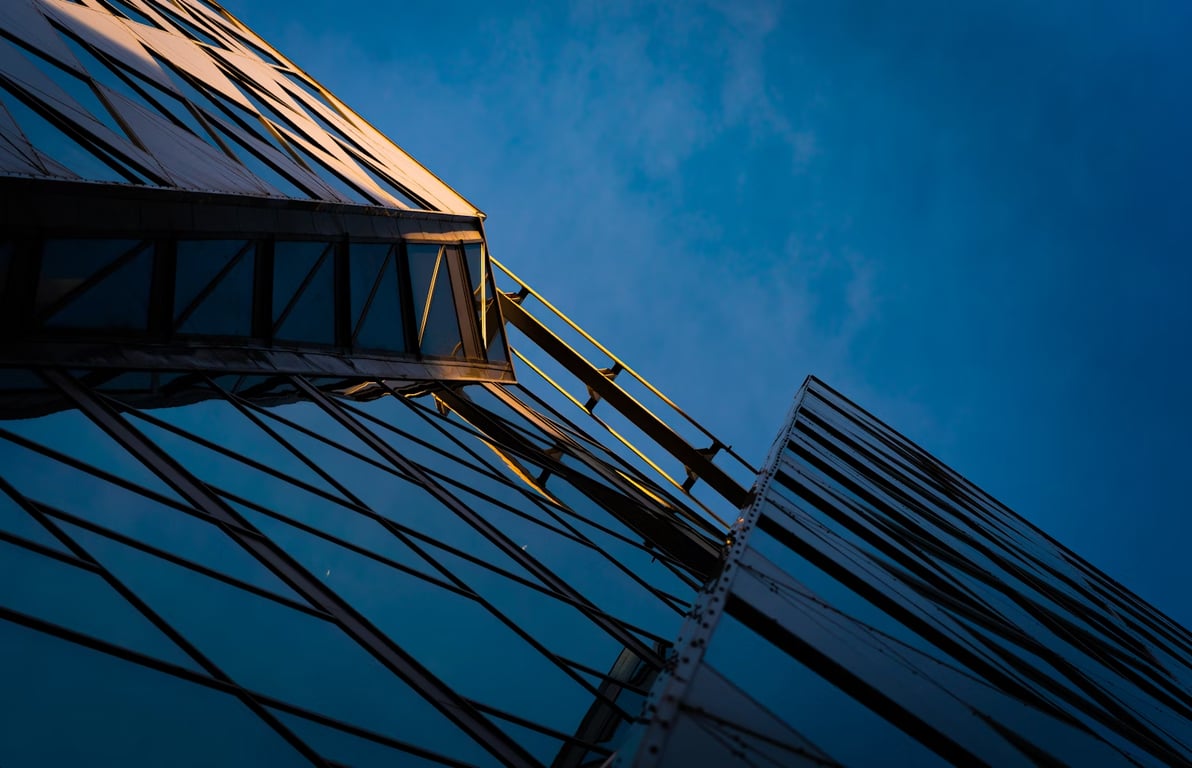
Photo by Jahanzeb Ahsan on Unsplash
When working with data in Python, pandas is often the first choice for data manipulation and analysis. Its powerful data structures, like DataFrame
and Series
, make it easy to handle and analyze large datasets. However, as the size of the data increases, pandas can become slow and memory-intensive, especially when dealing with datasets that do not fit entirely into memory. This is where dask, a flexible parallel computing library, comes into play. Dask provides pandas-like syntax for handling larger-than-memory datasets and leverages parallel processing to speed up computation.
In this blog post, we will explore how to handle large data files with pandas and dask, discussing the differences between the two and providing real-world examples of when and how to use them effectively.
1. Introduction: Working with Large Data Files
In today's data-driven world, large datasets are common. Whether you are analyzing customer behavior, processing scientific data, or working on a machine learning project, the data you work with may not always fit in memory. Handling these large datasets efficiently is a key challenge. Fortunately, Python offers several tools for working with large data files, and two of the most popular are pandas and dask.
pandas: Known for its user-friendly interface and powerful data structures, pandas is great for data analysis tasks but has some limitations when working with very large datasets.
dask: Designed for parallel computing, dask is an excellent choice when your data is too large to fit into memory, or when you want to speed up computations by distributing the workload across multiple cores or machines.
In this blog, we will compare both libraries and discuss the scenarios where each excels.
2. Challenges with Large Data Files in Python
Before we dive into the specifics of pandas and dask, it's important to understand some common challenges when working with large data files:
Memory Constraints: A single large dataset might not fit entirely into memory, leading to crashes or slow performance.
Long Processing Times: Processing large files can take a long time, especially when performing complex operations like aggregations, merges, or groupings.
I/O Bottlenecks: Reading and writing large files, particularly from disk or over a network, can be slow and inefficient.
To handle these challenges, it’s essential to adopt techniques that can scale with data size while keeping memory usage under control and speeding up processing.
3. pandas for Data Manipulation
What is pandas?
pandas is one of the most widely used libraries for data manipulation in Python. It provides data structures such as DataFrame
and Series
, which make it easy to handle structured data like tables, CSV files, and SQL queries.
How pandas Handles Data
pandas loads data into memory using DataFrame
objects, which are typically stored as 2-dimensional tables (like Excel spreadsheets) with rows and columns. This in-memory model works well for small to medium-sized datasets, where the entire dataset can fit into the system’s RAM.
import pandas as pd
# Load a CSV file into a pandas DataFrame
df = pd.read_csv('large_file.csv')
# Display the first few rows
print(df.head())
However, as the data size grows, pandas may struggle with memory efficiency. For example, when working with datasets larger than the available memory, pandas may cause your system to crash or severely slow down, as it tries to load the entire dataset into memory.
4. dask: A Scalable Solution for Large Data
What is dask?
dask is a flexible parallel computing library designed to scale Python code and handle large datasets. It integrates seamlessly with pandas and numpy, allowing you to perform computations in parallel and out-of-core (i.e., using disk storage when the dataset doesn’t fit in memory).
dask provides an API that mimics pandas, making it easy for users familiar with pandas to transition to dask when dealing with large data files. It uses lazy evaluation, meaning that operations are only executed when explicitly triggered, allowing for optimization and efficient resource management.
How dask Handles Large Data
dask’s core concept is the dask dataframe, which is similar to a pandas dataframe but operates in chunks. Each chunk is a pandas dataframe, and dask works on these chunks in parallel, making it possible to work with datasets that are too large to fit into memory.
Example: Loading a CSV File with daskimport dask.dataframe as dd
# Load a CSV file into a dask DataFrame
df = dd.read_csv('large_file.csv')
# Display the first few rows
print(df.head())
Notice that when using dask, you don't directly load the entire dataset into memory. Instead, dask reads the data in chunks and operates lazily on these chunks.
5. When to Use pandas vs. dask
Both pandas and dask are great tools for data analysis, but they serve different purposes and are suited for different types of tasks:
Use pandas when:
The data fits in memory (i.e., it is not too large).
You need to perform in-memory operations on the data.
You don’t need parallel processing or distributed computing.
Use dask when:
The data is too large to fit into memory.
You need parallel processing or out-of-core computations.
You need to scale your computations to multiple cores or even across a cluster of machines.
In short, if you are working with large datasets or need to scale your computations, dask is the better option. For smaller datasets, pandas is typically more efficient and easier to use.
6. Best Practices for Handling Large Data Files
6.1. Optimizing pandas for Large Data
If you decide to stick with pandas for large data, there are several optimization techniques you can use to reduce memory usage and improve performance:
Load Data in Chunks: Instead of loading the entire dataset into memory at once, load it in smaller chunks.
chunk_size = 100000
for chunk in pd.read_csv('large_file.csv', chunksize=chunk_size):
process(chunk)
Use
dtype
to Specify Data Types: By default, pandas may use more memory than necessary for data types. You can manually specifydtype
to optimize memory usage.
dtypes = {'column1': 'float32', 'column2': 'int8'}
df = pd.read_csv('large_file.csv', dtype=dtypes)
Use
usecols
to Read Specific Columns: If you only need a subset of columns, specify them with theusecols
parameter to save memory.
df = pd.read_csv('large_file.csv', usecols=['column1', 'column2'])
6.2. Using dask to Work with Larger-than-Memory Datasets
When working with datasets that don't fit into memory, dask’s ability to process data in parallel and out-of-core makes it an excellent choice. Dask breaks the data into smaller pieces and processes them in parallel, making computations much faster.
Lazy Loading: dask reads the data lazily, which means it does not load all data into memory at once. You can perform operations on the data without worrying about memory constraints.
Chunked Computation: With dask, computations are done in chunks, which allows you to process large datasets without exceeding memory limits.
6.3. Parallelizing Computations with dask
One of the major benefits of dask is its ability to parallelize computations. You can distribute your workload across multiple cores or even multiple machines.
# Use dask's `compute` function to trigger computation
df_result = df.groupby('column1').agg({'column2': 'mean'}).compute()
The compute()
function triggers the actual computation, which is processed in parallel across the available resources. This significantly speeds up data processing.
7. Examples: Working with Large Data Files Using pandas and dask
7.1. Loading Large CSV Files with pandas
import pandas as pd
# Load a large CSV file in chunks
chunk_size = 100000
chunks = pd.read_csv('large_file.csv', chunksize=chunk_size)
# Process each chunk
for chunk in chunks:
print(chunk.head())
7.2. Using dask to Load Large Data and Parallelize Operations
import dask.dataframe as dd
# Load a large CSV file with dask
df = dd.read_csv('large_file.csv')
# Perform a computation
result = df.groupby('column1').agg({'column2': 'mean'}).compute()
print(result)
8. Conclusion: Which Library Should You Use?
When handling large data files, pandas and dask both have their strengths. pandas is a go-to library for data manipulation when the data fits into memory, and it is easier to use for smaller datasets. However, for large datasets that don't fit into memory, dask provides an excellent solution by enabling parallel processing and allowing you to work with out-of-core data.
In summary:
Use pandas when dealing with data that fits in memory.
Switch to dask when working with larger-than-memory datasets, or when you need to perform parallel computations.
With these tools at your disposal, you can efficiently handle large data files in Python and scale your data processing as needed.
Happy coding!