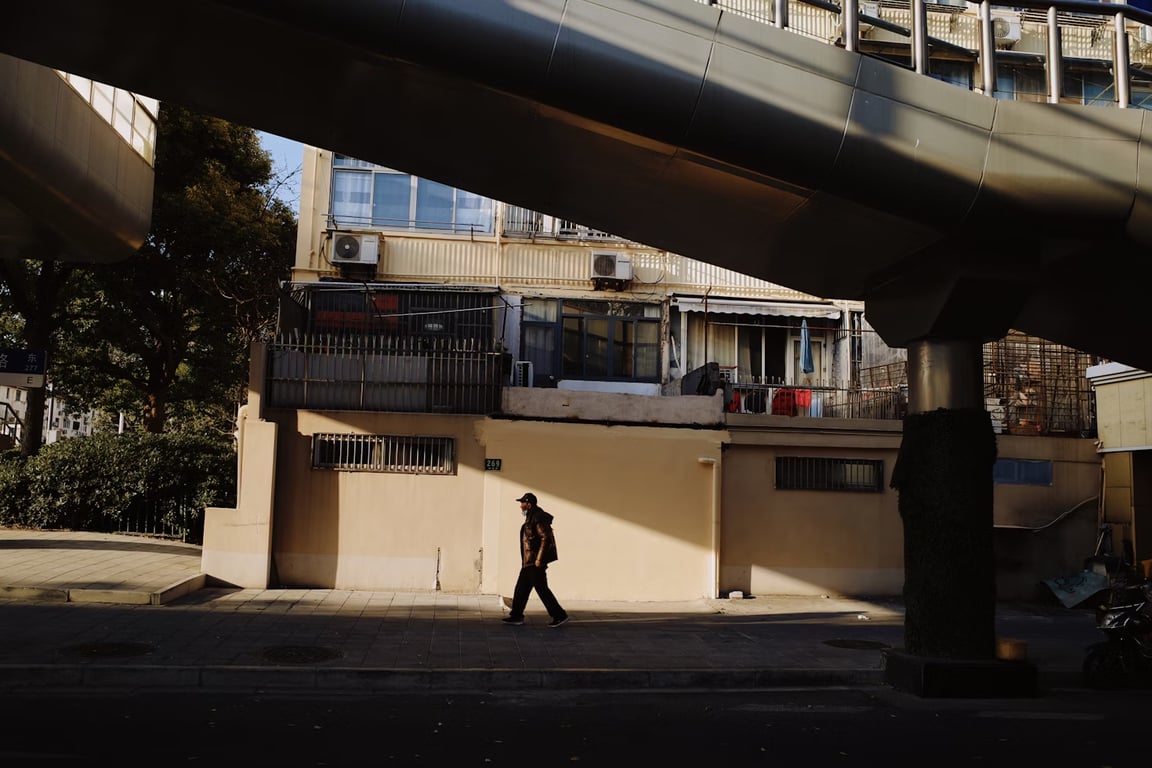
Photo by Angelina Yan on Unsplash
In today’s data-driven world, interactive dashboards have become indispensable tools for visualizing and analyzing data in real time. Dashboards provide a user-friendly way to summarize complex datasets and deliver actionable insights. Among the many Python libraries for creating visualizations, Plotly stands out for its ability to create dynamic, high-quality, and interactive dashboards.
In this blog, we will explore how to build interactive dashboards using Python’s Plotly library. By the end, you will have a clear understanding of Plotly’s features, how to set up your environment, and how to create an interactive dashboard step by step.
Table of Contents
What is Plotly?
Why Use Plotly for Dashboards?
Setting Up Your Environment
Plotly Basics: Getting Started with Visualizations
Introduction to Dash: Building Dashboards with Plotly
Step-by-Step Guide to Building an Interactive Dashboard
6.1. Data Preparation
6.2. Creating Visualizations
6.3. Building the Layout
6.4. Adding Interactivity
6.5. Running the Dashboard
Examples of Interactive Dashboards
Best Practices for Building Dashboards
Conclusion
1. What is Plotly?
Plotly is a versatile Python library that provides tools for creating interactive and high-quality visualizations. It supports a wide range of chart types, including line plots, bar charts, scatter plots, heatmaps, 3D charts, and more. The library is particularly well-suited for building dashboards when paired with Dash, a framework that enables the creation of interactive web-based applications.
2. Why Use Plotly for Dashboards?
Plotly has several features that make it a preferred choice for creating dashboards:
Interactivity: Plotly charts are interactive by default, allowing users to zoom, pan, and hover over data points.
Web-Based: Dashboards built with Plotly can be deployed as web applications accessible through a browser.
Cross-Library Integration: Plotly integrates seamlessly with pandas, NumPy, and other Python libraries.
Customization: The library provides extensive options for customizing plots and layouts.
Open Source: Plotly is free and open-source, with advanced features available through Plotly’s commercial offerings.
3. Setting Up Your Environment
Before creating dashboards, you need to install Plotly and Dash. Use the following commands to set up your Python environment:
pip install plotly dash pandas
Once installed, you’re ready to start creating visualizations and building dashboards.
4. Plotly Basics: Getting Started with Visualizations
Plotly makes it easy to create interactive visualizations. Here’s a quick example of a simple bar chart:
import plotly.graph_objects as go
# Sample data
x = ['Product A', 'Product B', 'Product C']
y = [20, 14, 23]
# Create a bar chart
fig = go.Figure(go.Bar(x=x, y=y))
fig.update_layout(title="Sales by Product", xaxis_title="Product", yaxis_title="Sales")
fig.show()
This script generates an interactive bar chart, which can be panned, zoomed, and hovered over.
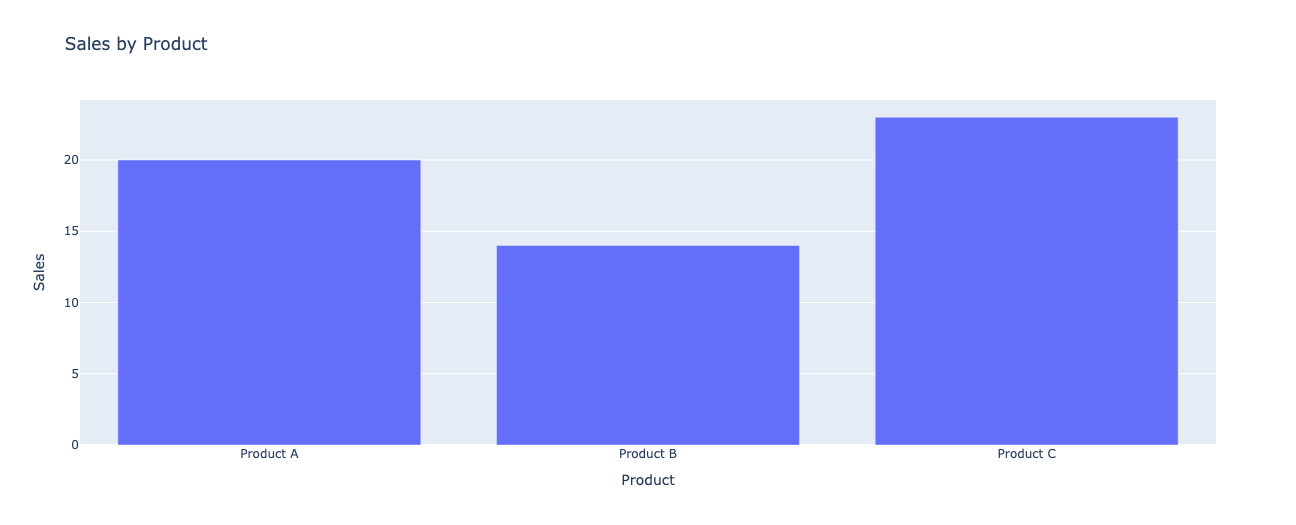
5. Introduction to Dash: Building Dashboards with Plotly
Dash is a Python framework built on top of Plotly that enables the creation of interactive dashboards. Dash applications are composed of two main parts:
Layout: Defines the structure and appearance of the dashboard.
Callbacks: Adds interactivity to the dashboard by connecting user inputs to outputs.
A basic Dash application looks like this:
from dash import Dash, html, dcc
app = Dash(__name__)
app.layout = html.Div([
html.H1("Hello Dash"),
dcc.Graph(
id='example-graph',
figure={
'data': [{'x': [1, 2, 3], 'y': [4, 1, 2], 'type': 'bar', 'name': 'Sample Data'}],
'layout': {'title': 'Example Plot'}
}
)
])
if __name__ == '__main__':
app.run_server(debug=True)
Running this script launches a local web server, and you can view the dashboard in your browser.
6. Step-by-Step Guide to Building an Interactive Dashboard
Let’s build a simple interactive dashboard using Plotly and Dash.
6.1. Data Preparation
First, gather and prepare your data. In this example, we’ll use a sample dataset about sales.
import pandas as pd
# Sample dataset
data = {
'Month': ['January', 'February', 'March', 'April', 'May'],
'Sales': [200, 220, 250, 270, 300],
'Expenses': [150, 160, 170, 180, 190]
}
df = pd.DataFrame(data)
6.2. Creating Visualizations
Use Plotly to create visualizations based on the dataset.
import plotly.express as px
# Create a line chart
sales_fig = px.line(df, x='Month', y='Sales', title='Monthly Sales')
expenses_fig = px.line(df, x='Month', y='Expenses', title='Monthly Expenses')
6.3. Building the Layout
Define the layout of your dashboard using Dash components.
from dash import html, dcc
app.layout = html.Div([
html.H1("Sales Dashboard"),
dcc.Graph(id='sales-graph', figure=sales_fig),
dcc.Graph(id='expenses-graph', figure=expenses_fig)
])
6.4. Adding Interactivity
Add interactivity by using Dash callbacks. For example, you can create a dropdown to filter data.
from dash import Input, Output
app.layout = html.Div([
html.H1("Sales Dashboard"),
dcc.Dropdown(
id='data-selector',
options=[
{'label': 'Sales', 'value': 'Sales'},
{'label': 'Expenses', 'value': 'Expenses'}
],
value='Sales'
),
dcc.Graph(id='line-chart')
])
@app.callback(
Output('line-chart', 'figure'),
[Input('data-selector', 'value')]
)
def update_chart(selected_data):
return px.line(df, x='Month', y=selected_data, title=f'Monthly {selected_data}')
6.5. Running the Dashboard
Run your dashboard using the run_server
method.
if __name__ == '__main__':
app.run_server(debug=True)
7. Examples of Interactive Dashboards
Here are some examples of dashboards you can build with Plotly and Dash:
Sales Performance Dashboard: Tracks revenue, expenses, and profit trends.
Customer Analytics Dashboard: Displays customer demographics, behavior, and segmentation.
Website Traffic Dashboard: Analyzes traffic sources, page views, and bounce rates.
8. Best Practices for Building Dashboards
To create effective dashboards, follow these best practices:
Define Your Objectives: Clearly define the purpose of the dashboard and the key metrics it should display.
Keep It Simple: Avoid clutter and focus on the most important visualizations.
Optimize Performance: Use efficient data queries and avoid overloading the dashboard with too many visualizations.
Make It Responsive: Ensure your dashboard looks good on different devices and screen sizes.
Provide Context: Add titles, labels, and tooltips to make your visualizations easy to understand.
9. Conclusion
Plotly and Dash make it easy to build beautiful and interactive dashboards using Python. By leveraging their features, you can create tools that provide actionable insights, enhance decision-making, and impress stakeholders. Whether you're tracking sales, analyzing customer behavior, or monitoring system performance, Plotly and Dash can help you bring your data to life.
Start experimenting with these tools today and take your data visualization skills to the next level.
Happy coding!