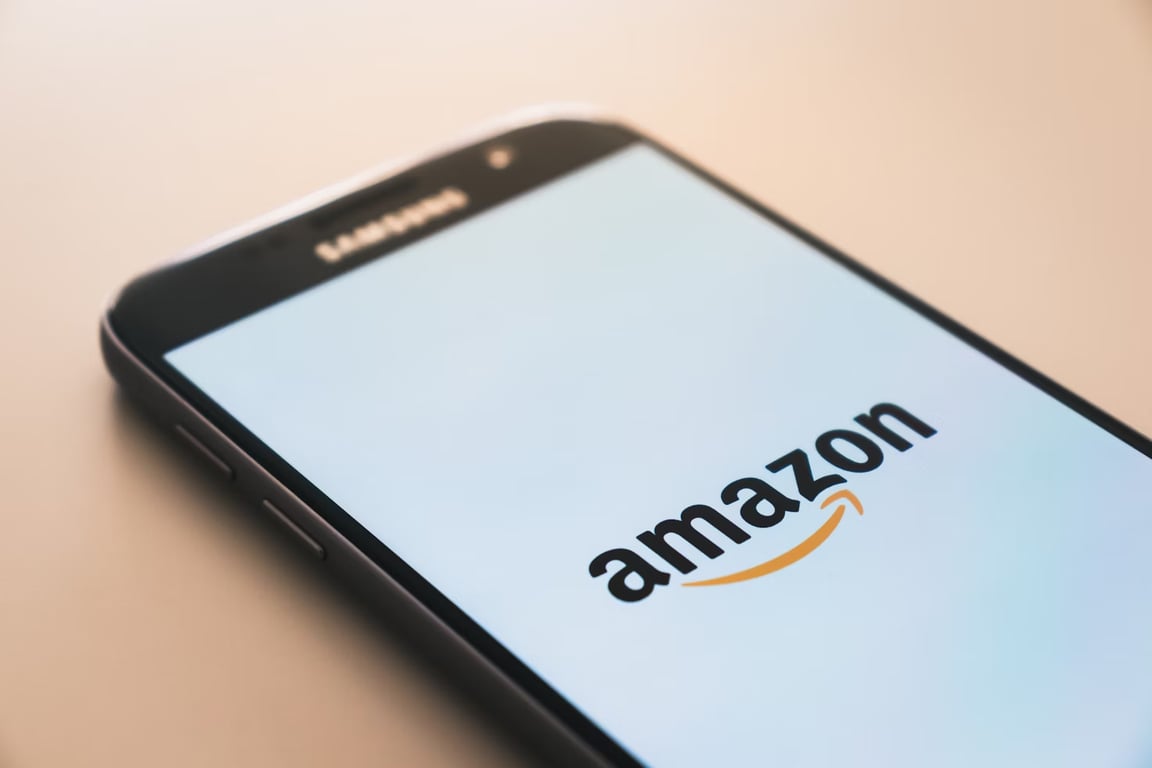
Photo by Christian Wiediger on Unsplash
The serverless architecture is gaining significant popularity for its ability to simplify the deployment and management of applications. It allows developers to focus solely on writing code without worrying about the underlying infrastructure. AWS Lambda and Zappa are two powerful tools that enable serverless Python development. In this blog, we'll walk through the basics of serverless computing, explore how AWS Lambda and Zappa work with Python, and provide a step-by-step guide to building and deploying a serverless Python application.
Table of Contents
What is Serverless Computing?
Benefits of Serverless Architecture
What is AWS Lambda?
What is Zappa?
Setting Up AWS Lambda for Python Applications
Deploying Python Applications to AWS Lambda
Using Zappa to Deploy Python Applications
Real-World Example: Building a Serverless API with AWS Lambda and Zappa
Best Practices for Serverless Python Development
Conclusion
1. What is Serverless Computing?
Serverless computing is an architecture where cloud providers manage the infrastructure, allowing developers to focus only on writing code. It eliminates the need for provisioning, managing, and scaling servers. The term "serverless" doesn’t mean there are no servers involved; rather, it means the cloud provider takes care of all the server management tasks, enabling developers to run their code without explicitly dealing with the underlying hardware.
Key Features of Serverless Computing:
Event-driven: Serverless applications are typically event-driven, meaning they execute in response to specific events such as HTTP requests, database changes, or file uploads.
Automatic Scaling: Serverless services automatically scale up or down based on demand. There’s no need to worry about overprovisioning or underprovisioning resources.
Cost Efficiency: In a serverless setup, you only pay for the actual compute time your code uses. There’s no need to pay for idle servers.
Managed Infrastructure: The cloud provider manages everything from the operating system to the runtime environment.
2. Benefits of Serverless Architecture
Serverless architecture offers several key benefits for Python developers:
Reduced Operational Complexity: Developers don’t need to worry about managing servers, operating systems, or scaling resources.
Cost Savings: You only pay for the compute resources your application uses. This model is especially beneficial for applications with unpredictable or low traffic.
Faster Time to Market: Serverless allows developers to focus on writing the core functionality without managing infrastructure, speeding up development cycles.
Scalability: Serverless platforms like AWS Lambda automatically scale your application based on demand, ensuring that you can handle high volumes of traffic with minimal configuration.
Improved Reliability: Cloud providers offer high availability and fault tolerance, so serverless applications tend to be more reliable.
3. What is AWS Lambda?
AWS Lambda is a serverless compute service that lets you run code without provisioning or managing servers. Lambda executes your code only when needed and scales automatically to handle requests. It integrates seamlessly with other AWS services, making it easy to build a serverless architecture.
Key Features of AWS Lambda:
Event-driven: Lambda functions are invoked by specific events, such as HTTP requests via API Gateway or changes in AWS S3 buckets.
Language Support: Lambda supports multiple languages, including Python, Node.js, Java, and C#.
Automatic Scaling: AWS Lambda automatically scales based on the number of incoming requests.
Pay-as-you-go Model: You are charged based on the number of requests and the time your code runs (measured in milliseconds).
Common Use Cases for AWS Lambda:
Web APIs: Building and deploying RESTful APIs.
Data Processing: Processing files uploaded to S3 or stream data.
Real-time Image or Video Processing: Resizing or transcoding media files upon upload.
Automation: Automating various tasks based on events, such as cleaning up old data or sending notifications.
4. What is Zappa?
Zappa is a Python library that simplifies the deployment of Python web applications to AWS Lambda and API Gateway. It allows you to quickly deploy and manage Python web apps, making it easier to set up serverless applications.
Zappa automates the process of creating the necessary Lambda functions, API Gateway configurations, and CloudFormation stacks for you. It’s particularly useful for deploying Flask and Django applications to AWS Lambda.
Key Features of Zappa:
Flask and Django Support: Zappa works seamlessly with Flask and Django, enabling you to deploy these web frameworks on AWS Lambda.
Automatic Configuration: Zappa handles the configuration of API Gateway and Lambda functions, which can be complex to manage manually.
Scalable: Zappa takes full advantage of AWS Lambda's scaling features.
Built-in Management Tools: Zappa provides simple commands for deploying, managing, and monitoring your application.
5. Setting Up AWS Lambda for Python Applications
To get started with AWS Lambda and Python, follow these steps:
5.1. Create an AWS Account
If you don’t already have an AWS account, sign up at AWS's official site.
5.2. Create a Lambda Function
Log into AWS Console: Navigate to the AWS Lambda section in the AWS Management Console.
Create a New Function: Choose the "Author from Scratch" option. Give your function a name (e.g.,
hello_world
) and select Python as the runtime.Set Permissions: Attach an IAM role to your Lambda function with the necessary permissions. If you're just experimenting, you can use the default execution role.
5.3. Write Your Lambda Function
In the AWS Lambda console, you can write your Python function directly. Here's a basic example of a "Hello, World!" Lambda function:
def lambda_handler(event, context):
return {
'statusCode': 200,
'body': 'Hello, World!'
}
5.4. Test the Function
You can create test events within the AWS Lambda console and invoke the function. You’ll receive the output in the response section.
6. Deploying Python Applications to AWS Lambda
You can deploy more complex Python applications to AWS Lambda by packaging your code and dependencies into a deployment package. However, for simplicity, we’ll use Zappa to automate the deployment process.
7. Using Zappa to Deploy Python Applications
Zappa streamlines the process of deploying Python web applications, especially Flask and Django apps, to AWS Lambda.
7.1. Install Zappa
Start by installing Zappa:
pip install zappa
7.2. Initialize a Zappa Project
For a Flask application, create a new directory and initialize a Flask app:
mkdir zappa_project
cd zappa_project
pip install Flask
touch app.py
In app.py
, add the following simple Flask app:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello():
return "Hello, Serverless World!"
if __name__ == '__main__':
app.run()
Next, run the Zappa initialization command:
zappa init
This command will prompt you to select your AWS region and set up your Zappa project configuration. It will also create a zappa_settings.json
file.
7.3. Deploy the Application
Once everything is set up, deploy the Flask app using the following command:
zappa deploy production
Zappa will create the necessary Lambda function and API Gateway configuration for you.
7.4. Test the Application
After deployment, you’ll get a URL from Zappa that you can use to access your application. You can open this URL in your browser to see your serverless Flask application in action.
8. Real-World Example: Building a Serverless API with AWS Lambda and Zappa
Let’s take the example of a serverless REST API that provides a list of users. We'll build this API using Flask and deploy it with Zappa.
Create the Flask App:
In app.py
, add the following code:
from flask import Flask, jsonify
app = Flask(__name__)
users = [
{"id": 1, "name": "John Doe"},
{"id": 2, "name": "Jane Doe"}
]
@app.route('/users', methods=['GET'])
def get_users():
return jsonify(users)
if __name__ == '__main__':
app.run()
Deploy with Zappa:
After setting up Zappa with zappa init
, deploy the app:
zappa deploy production
Test the API:
Once deployed, use the provided URL to access your API and retrieve the list of users.
9. Best Practices for Serverless Python Development
Efficient Resource Management: Make sure your Lambda functions are optimized for performance to avoid unnecessary costs.
Use API Gateway: For web APIs, always use API Gateway in conjunction with Lambda to manage routing and access.
Manage Dependencies: Keep your deployment package lean and only include the dependencies required for your Lambda function.
Monitor and Debug: Use AWS CloudWatch to log and monitor your Lambda functions for troubleshooting.
10. Conclusion
Serverless Python development with AWS Lambda and Zappa provides a powerful way to build scalable, efficient, and cost-effective applications. While AWS Lambda handles the backend infrastructure, Zappa simplifies the deployment process, especially for Flask and Django applications. Whether you're building a simple API or a complex data processing application, serverless computing can save time, reduce operational overhead, and allow you to focus on building features rather than managing infrastructure.
Happy coding!