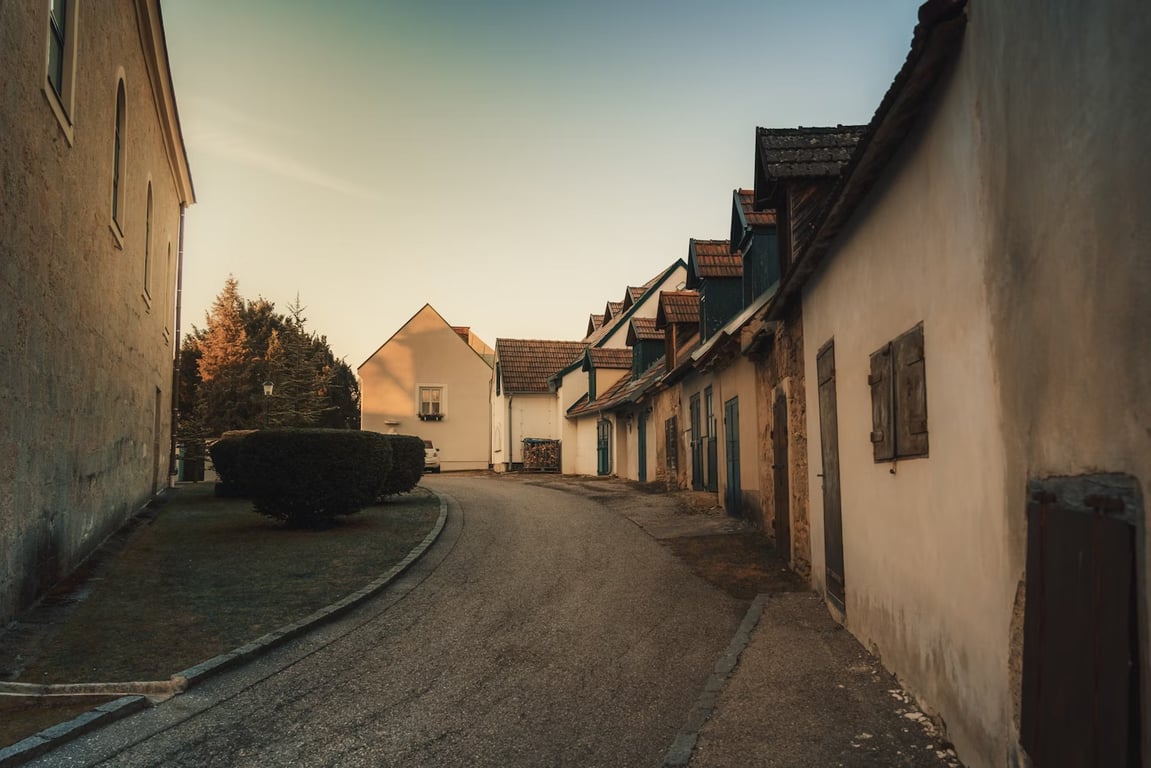
Photo by Michael Pointner on Unsplash
Building RESTful APIs is a crucial aspect of modern web development. They allow different applications to communicate over the web, making them an essential tool for building scalable and robust applications. In Python, two of the most popular frameworks for building REST APIs are Flask and FastAPI. This blog will provide an in-depth guide on building REST APIs using both Flask and FastAPI. We will explore their features, installation, and basic usage to help you decide which framework is right for your project.
Table of Contents
Introduction to REST APIs
Why Choose Flask and FastAPI?
Setting Up Flask for REST API Development
Creating a REST API with Flask
Setting Up FastAPI for REST API Development
Creating a REST API with FastAPI
Performance Comparison: Flask vs. FastAPI
Conclusion
1. Introduction to REST APIs
REST (Representational State Transfer) is an architectural style for designing networked applications. It relies on stateless communication and uses standard HTTP methods such as GET, POST, PUT, DELETE, and PATCH for operations.
In a RESTful API, resources are represented by URLs, and HTTP methods define actions on those resources. For example:
GET /users
– Retrieves a list of usersPOST /users
– Creates a new userPUT /users/{id}
– Updates a userDELETE /users/{id}
– Deletes a user
REST APIs are commonly used in web and mobile applications to enable communication between the client and server.
2. Why Choose Flask and FastAPI?
2.1 Flask
Flask is a lightweight, flexible, and minimalistic web framework for Python. It’s perfect for small to medium-sized projects where you want to quickly prototype and build a simple REST API. Some of Flask's key features include:
Simple to learn and use
Minimalistic design (doesn't force you to use certain tools or libraries)
Flexible and extensible with third-party libraries
Great community support
2.2 FastAPI
FastAPI is a modern, fast, and highly efficient web framework for building APIs. It is built on top of Python’s asynchronous features and is designed to help you create APIs quickly with minimal effort. Some of its notable features include:
Extremely fast performance (due to Starlette and Pydantic)
Asynchronous support for better concurrency
Automatic generation of OpenAPI and JSON Schema documentation
Built-in validation using Pydantic models
Type hints for more readable and reliable code
3. Setting Up Flask for REST API Development
To begin building a REST API with Flask, you’ll first need to install Flask. You can install it using pip:
pip install Flask
3.1. Basic Flask Application Structure
Create a new directory for your project and create a Python file (e.g., app.py
):
mkdir flask_rest_api
cd flask_rest_api
touch app.py
In app.py
, import Flask and create a basic Flask app:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api', methods=['GET'])
def home():
return jsonify({"message": "Welcome to the Flask API!"})
if __name__ == '__main__':
app.run(debug=True)
To run the application, execute
python app.py
Now, you can visit http://127.0.0.1:5000/api
in your browser or Postman, and it should display the message: {"message": "Welcome to the Flask API!"}
.
4. Creating a REST API with Flask
4.1. Define a Simple API for Managing Users
Let’s build a simple REST API that manages a list of users. In a real-world application, you would interact with a database, but for simplicity, we will use an in-memory list of users.
Here’s the code to implement basic GET
, POST
, and DELETE
endpoints:
from flask import Flask, jsonify, request
app = Flask(__name__)
# Sample data
users = [
{"id": 1, "name": "John Doe"},
{"id": 2, "name": "Jane Doe"}
]
@app.route('/api/users', methods=['GET'])
def get_users():
return jsonify(users)
@app.route('/api/users', methods=['POST'])
def create_user():
new_user = request.get_json()
users.append(new_user)
return jsonify(new_user), 201
@app.route('/api/users/<int:user_id>', methods=['DELETE'])
def delete_user(user_id):
global users
users = [user for user in users if user["id"] != user_id]
return '', 204
if __name__ == '__main__':
app.run(debug=True)
4.2. Explanation
GET /api/users
: Returns a list of all users.POST /api/users
: Accepts a JSON body to create a new user and adds it to theusers
list.DELETE /api/users/{id}
: Deletes a user by their ID.
You can test these endpoints using tools like Postman or cURL.
5. Setting Up FastAPI for REST API Development
FastAPI can be installed using pip:
pip install fastapi uvicorn
FastAPI uses Uvicorn as the ASGI server for running the application, so it needs to be installed as well.
5.1. Basic FastAPI Application Structure
Create a new directory for your FastAPI project:
mkdir fastapi_rest_api
cd fastapi_rest_api
touch main.py
In main.py
, create a basic FastAPI application:
from fastapi import FastAPI
app = FastAPI()
@app.get("/api")
def read_root():
return {"message": "Welcome to the FastAPI API!"}
To run the application, execute:
uvicorn main:app --reload
Now, you can visit http://127.0.0.1:8000/api
in your browser, and you should see the message: {"message": "Welcome to the FastAPI API!"}
.
6. Creating a REST API with FastAPI
6.1. Define a Simple API for Managing Users
We will now implement the same user management API that we built with Flask earlier, but this time using FastAPI.
from fastapi import FastAPI, HTTPException
from pydantic import BaseModel
app = FastAPI()
# Sample data
users = [
{"id": 1, "name": "John Doe"},
{"id": 2, "name": "Jane Doe"}
]
class User(BaseModel):
id: int
name: str
@app.get("/api/users")
def get_users():
return users
@app.post("/api/users")
def create_user(user: User):
users.append(user.dict())
return user, 201
@app.delete("/api/users/{user_id}")
def delete_user(user_id: int):
global users
users = [user for user in users if user["id"] != user_id]
return {"message": "User deleted successfully"}
6.2. Explanation
GET /api/users
: Returns a list of all users.POST /api/users
: Accepts a JSON body to create a new user and adds it to theusers
list.DELETE /api/users/{id}
: Deletes a user by their ID.
The use of Pydantic’s BaseModel
provides automatic validation of the incoming data, ensuring that the user data adheres to the specified format.
7. Performance Comparison: Flask vs. FastAPI
7.1. Flask
Flask is synchronous, meaning each request is processed one at a time. While Flask is suitable for smaller applications and simpler projects, it may not perform as well under heavy load or when handling many concurrent requests.
7.2. FastAPI
FastAPI, on the other hand, is asynchronous and designed to handle high concurrency. It performs exceptionally well in terms of speed, especially for I/O-bound tasks. FastAPI’s async capabilities are built on top of Python's asyncio
library, allowing it to handle multiple requests simultaneously, which gives it a significant performance advantage.
In benchmarks, FastAPI has been shown to be one of the fastest Python frameworks, often outperforming Flask and other web frameworks.
8. Conclusion
In this guide, we explored how to build REST APIs using Flask and FastAPI. Both frameworks are excellent choices, but they suit different needs:
Flask is ideal for small to medium-sized applications where simplicity, flexibility, and ease of use are priorities.
FastAPI is perfect for larger, high-performance applications where speed, asynchronous processing, and automatic validation are essential.
Ultimately, your choice of framework will depend on your specific requirements and the scale of your project. Both Flask and FastAPI are powerful and capable tools for building robust APIs in Python.
Happy coding!