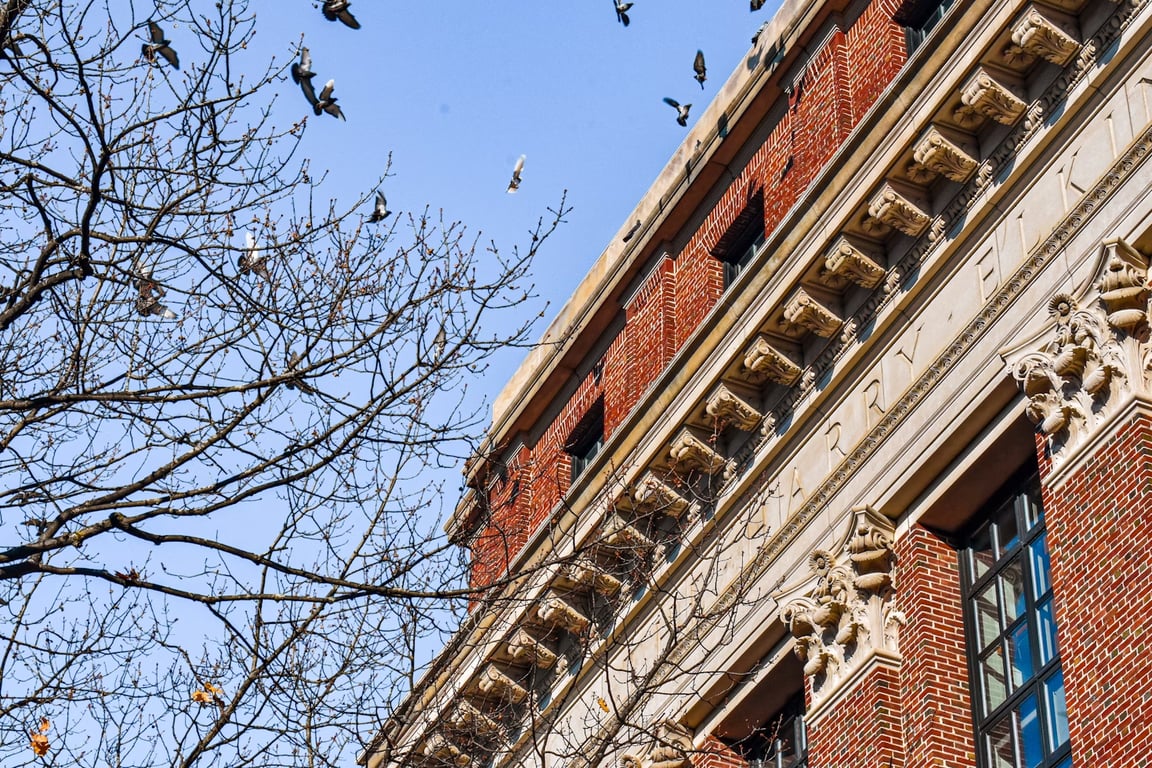
Data pipelines are essential components of modern software applications, especially in data processing and machine learning workflows. These pipelines efficiently move data from one stage to the next, often involving transformations and various types of data processing. In Python, you can create powerful and efficient data pipelines using generators and coroutines. These features allow for lazy evaluation, asynchronous processing, and memory-efficient handling of large data sets.
In this blog, we’ll explore how to create data pipelines in Python with the help of generators and coroutines, highlighting the key concepts, their benefits, and practical examples to help you build your own data pipelines.
What is a Data Pipeline?
A data pipeline is a series of processing steps that data undergoes from its raw form to its final output, such as being stored, visualized, or used for analysis. In simple terms, it’s a sequence of operations that transform data into useful information.
A basic data pipeline involves the following steps:
Data Ingestion: Reading data from various sources like APIs, databases, or files.
Data Transformation: Cleaning, filtering, and transforming the data.
Data Loading: Storing or using the transformed data.
In this blog, we’ll be focusing on how to implement the "transformation" step efficiently using Python’s generators and coroutines.
Generators: A Lazy Approach to Data Processing
What Are Python Generators?
Generators are a special type of iterator in Python that allow you to iterate over data without needing to load everything into memory at once. They are defined using functions with the yield
keyword. Unlike traditional functions, which return a single value, a generator yields multiple values one at a time, suspending execution between yields.
The key benefit of using generators in data pipelines is that they support lazy evaluation. This means that they only compute the next value when needed, which is particularly useful when working with large datasets that cannot fit into memory at once.
How to Create a Generator in Python
Here’s a simple example of a generator that generates squares of numbers up to a given limit:
def square_numbers(n):
for i in range(n):
yield i * i
# Create a generator object
gen = square_numbers(5)
# Iterate over the generator
for num in gen:
print(num)
Output:
0
1
4
9
16
Each time the yield
keyword is encountered, the function suspends execution and returns the current value. When the generator is iterated again, it resumes from where it left off.
Why Use Generators in Data Pipelines?
Memory Efficiency: Since generators yield one item at a time, they don’t require storing all items in memory at once.
Lazy Evaluation: Data is processed only when required, which can significantly reduce computation time for large datasets.
Simplicity: Generators can simplify code by removing the need for manual iteration and bookkeeping.
Example: Simple Data Pipeline with Generators
Let’s create a simple data pipeline using generators that reads numbers from a file, squares them, and writes the results to another file.
def read_numbers(filename):
with open(filename, 'r') as file:
for line in file:
yield int(line.strip())
def square_numbers(numbers):
for number in numbers:
yield number * number
def write_results(filename, results):
with open(filename, 'w') as file:
for result in results:
file.write(f"{result}\n")
# Using the data pipeline
numbers = read_numbers('numbers.txt')
squared_numbers = square_numbers(numbers)
write_results('squared_numbers.txt', squared_numbers)
In this example:
read_numbers
reads integers from a file line by line.square_numbers
squares each number.write_results
writes the squared numbers to a new file.
By using generators, we process the numbers lazily, meaning that we don’t load the entire file into memory at once.
Coroutines: Building Asynchronous Pipelines
While generators provide a way to process data lazily, coroutines take it a step further by allowing for asynchronous programming. A coroutine is a special type of generator that can consume data asynchronously and perform non-blocking operations. Coroutines are typically used for tasks like handling I/O-bound operations (e.g., making network requests or interacting with databases).
What Are Python Coroutines?
Coroutines are defined using the async def
syntax. They allow for asynchronous execution and are typically used in combination with Python’s asyncio
module to create non-blocking tasks.
Coroutines use await
to pause their execution until a certain result is available. This makes coroutines ideal for situations where tasks are I/O-bound, as they can wait for one task to complete without blocking others.
Example: Using Coroutines for Data Ingestion
Suppose we are building a data pipeline that ingests data from multiple APIs concurrently. We can use coroutines to handle the I/O-bound API requests asynchronously.
import asyncio
import aiohttp
# Coroutine to fetch data from an API
async def fetch_data(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.json()
# Coroutine to process the fetched data
async def process_data(urls):
tasks = [fetch_data(url) for url in urls]
results = await asyncio.gather(*tasks)
return results
# Define the URLs to fetch data from
urls = ['https://api.example1.com', 'https://api.example2.com']
# Run the data pipeline asynchronously
async def run_pipeline():
data = await process_data(urls)
print(data)
# Run the event loop
asyncio.run(run_pipeline())
In this example, fetch_data
is a coroutine that asynchronously fetches data from an API. The process_data
coroutine launches multiple tasks concurrently, using asyncio.gather()
to run them in parallel.
When to Use Coroutines in Data Pipelines?
I/O-Bound Operations: Coroutines are useful when your data pipeline involves tasks that wait on I/O, such as making network requests, reading from databases, or accessing cloud storage.
Concurrent Execution: They allow multiple I/O-bound tasks to run concurrently, reducing overall pipeline runtime.
Non-blocking: Coroutines do not block the main thread, making them efficient for handling multiple tasks at once.
Combining Generators and Coroutines for Data Pipelines
You can also combine generators and coroutines to create more complex and efficient data pipelines. For example, you might want to use generators to process data lazily, while using coroutines to perform asynchronous I/O operations in parallel.
Example: Data Pipeline with Generators and Coroutines
Let’s say we have a pipeline that reads data from a file, fetches additional information from an API, and then writes the results to a file. We can combine both concepts to create a powerful pipeline.
import asyncio
import aiohttp
# Generator to read data from a file
def read_data(filename):
with open(filename, 'r') as file:
for line in file:
yield line.strip()
# Coroutine to fetch additional data from an API
async def fetch_additional_data(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.json()
# Generator to process and fetch data for each line in the file
async def process_data(filename, api_url):
for line in read_data(filename):
additional_data = await fetch_additional_data(api_url + line)
yield line, additional_data
# Write the processed data to a file
def write_results(filename, results):
with open(filename, 'w') as file:
for line, data in results:
file.write(f"{line}: {data}\n")
# Define the API URL
api_url = "https://api.example.com/data/"
# Run the pipeline
async def run_pipeline():
results = process_data('input.txt', api_url)
await write_results('output.txt', results)
# Execute the event loop
asyncio.run(run_pipeline())
In this example:
Generators: The
read_data
function reads lines from a file lazily.Coroutines: The
fetch_additional_data
function fetches data from an API asynchronously.Combined Pipeline: The
process_data
function combines these elements, processing each line lazily and concurrently fetching additional data from an API.
Benefits of Using Generators and Coroutines in Data Pipelines
Memory Efficiency: Generators allow you to handle large datasets without consuming too much memory, while coroutines help manage I/O-bound tasks without blocking the main thread.
Improved Performance: Coroutines enable you to execute multiple tasks concurrently, speeding up I/O-bound operations such as API calls and database queries.
Clean Code: Generators and coroutines make your code more readable and maintainable by separating the logic of data processing and asynchronous tasks.
Scalability: Both generators and coroutines are highly scalable, which makes them ideal for building data pipelines that can handle large volumes of data or high concurrency.
Conclusion
In this blog, we explored how to build efficient and scalable data pipelines in Python using generators and coroutines. Generators help us process data lazily and efficiently, while coroutines enable asynchronous execution of I/O-bound tasks. By combining these two features, we can create powerful data pipelines that are both memory-efficient and high-performance.
With Python's powerful async capabilities and lazy evaluation model, you can easily build pipelines that can scale with your data processing needs, making them ideal for modern data workflows in data science, machine learning, and web scraping applications.
Happy coding!