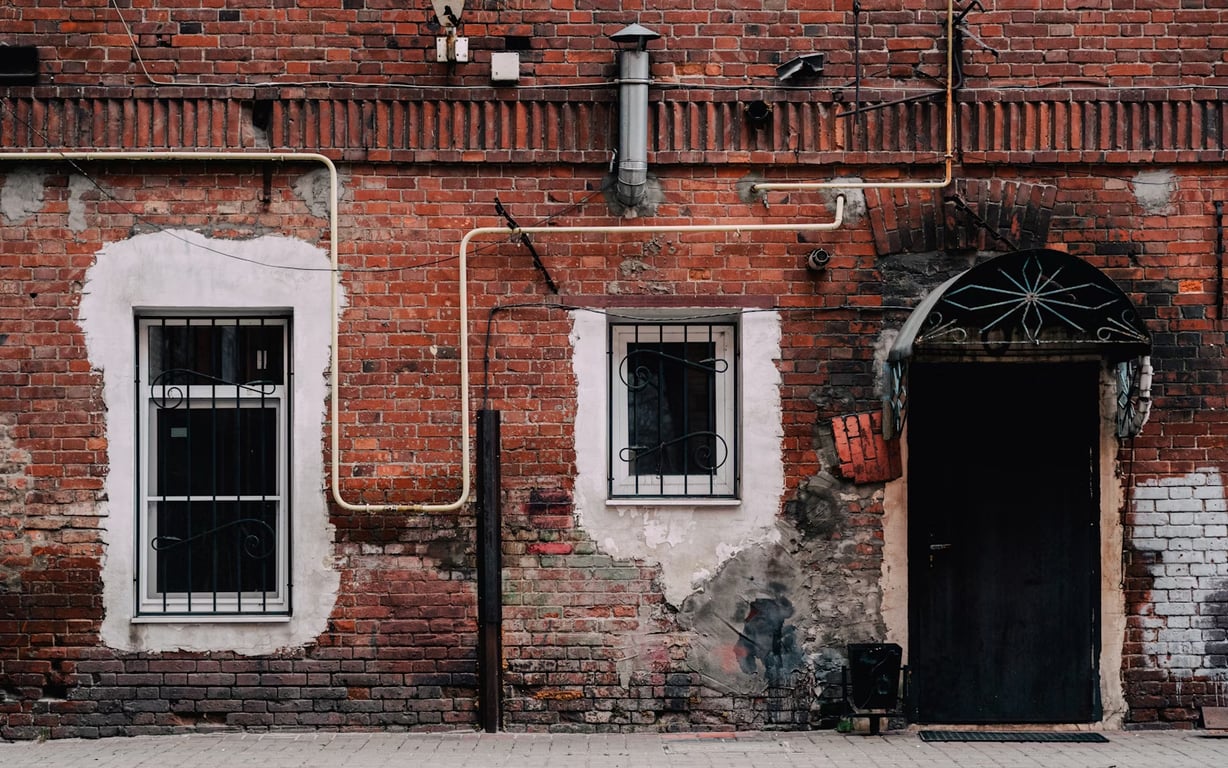
Photo by Yuriy Vertikov on Unsplash
In 2025, writing clean and Pythonic code remains a core principle for developers who want to produce efficient, readable, and maintainable software. Python, with its simplicity and readability, emphasizes code that is intuitive and easy to understand. But even with Python’s clean syntax, writing Pythonic code is an art that goes beyond just using the language’s syntax—it’s about adopting best practices that lead to clean, efficient, and maintainable code.
In this blog, we will explore the key principles and best practices for writing Pythonic code. We’ll cover aspects like code style, readability, and performance optimization, while also providing real-world examples to demonstrate how to implement these practices in your projects.
1. Introduction: What is Pythonic Code?
“Pythonic” is a term used to describe code that adheres to the principles of Python’s design philosophy. It emphasizes readability, simplicity, and using Python's features in a way that is natural to the language. Writing Pythonic code isn't just about following syntax; it’s about embracing Python's philosophy of clean, efficient, and maintainable programming.
The goal of writing Pythonic code is to make the codebase more understandable and easier to maintain. It involves selecting the right constructs, libraries, and techniques that are idiomatic to Python.
2. Why Writing Clean Python Code Matters
Writing clean and Pythonic code has many benefits:
Readability: Python's emphasis on readability makes it easy to read and understand code, even for people who aren't familiar with the specific project.
Maintainability: Clean code is easier to maintain and update. It reduces the chances of introducing bugs and ensures that modifications can be made without breaking existing functionality.
Collaboration: When multiple developers work on the same codebase, writing clean code ensures that everyone can follow the logic and contribute effectively.
Scalability: Pythonic code tends to be efficient and scalable, ensuring your application performs well as it grows.
Writing clean code is essential for long-term project success, especially in a language like Python, where simplicity is one of its core tenets.
3. The Zen of Python
Before diving into best practices, it’s essential to understand The Zen of Python. This collection of aphorisms, written by Tim Peters, captures Python's design principles. You can view it by running the following command in Python:
import this
Some key aphorisms to keep in mind when writing Pythonic code are:
"Readability counts."
"There should be one—and preferably only one—obvious way to do it."
"Simple is better than complex."
"Flat is better than nested."
These principles provide a foundation for writing Pythonic code that is clean, intuitive, and maintainable.
4. Adhering to PEP 8: The Style Guide for Python Code
PEP 8 (Python Enhancement Proposal 8) is the official style guide for Python code. It sets standards for indentation, naming conventions, line length, and other important factors. Adhering to PEP 8 ensures consistency in your codebase, making it easier for others to read and contribute.
Some important points from PEP 8 include:
Indentation: Use 4 spaces per indentation level. Never use tabs.
Line Length: Limit all lines to a maximum of 79 characters (72 for docstrings).
Naming Conventions:
Use lowercase with underscores for function and variable names (e.g.,
my_function
).Use CapitalizedWords for class names (e.g.,
MyClass
).Use ALL_CAPS with underscores for constants (e.g.,
MAX_SIZE
).
Blank Lines: Use blank lines to separate functions and classes, and to separate logical sections of the code.
By following PEP 8, you create code that aligns with Python's conventions, making it easier for others to read and maintain.
5. Best Practices for Writing Pythonic Code
5.1. Use Meaningful Variable and Function Names
The names of your variables and functions should clearly describe what they represent or what they do. Descriptive names improve the readability and understanding of the code.
Bad Example:
a = 10
b = 5
c = a + b
Good Example:
num_apples = 10
num_oranges = 5
total_fruits = num_apples + num_oranges
In the good example, the variable names clearly convey the meaning, making the code more readable.
5.2. Write Simple and Concise Code
Python emphasizes simplicity. Avoid unnecessary complexity and choose the most straightforward approach to solving a problem.
Bad Example:
def sum_of_squares(nums):
result = 0
for num in nums:
result += num ** 2
return result
Good Example:
def sum_of_squares(nums):
return sum(num ** 2 for num in nums)
The good example uses a Pythonic approach with a generator expression and the built-in sum()
function, making the code more concise and readable.
5.3. Embrace List Comprehensions and Generators
Python provides powerful tools like list comprehensions and generators to simplify your code and make it more efficient. List comprehensions offer a concise way to create lists, and generators allow for efficient lazy evaluation.
List Comprehension Example:
squares = [x ** 2 for x in range(10)]
Generator Example:
def generate_squares():
for x in range(10):
yield x ** 2
List comprehensions and generators are Pythonic ways to work with collections and make your code more expressive.
5.4. Avoid Using Explicit Loops When Not Necessary
When iterating over a collection, prefer Python's built-in functions and constructs over manually written loops. This leads to more concise and Pythonic code.
Bad Example:
squared_numbers = []
for num in range(10):
squared_numbers.append(num ** 2)
Good Example:
squared_numbers = [num ** 2 for num in range(10)]
The second approach is cleaner, more concise, and easier to read.
5.5. Use Python’s Built-in Functions and Libraries
Python provides a rich set of built-in functions and libraries that handle common tasks. Whenever possible, use these tools instead of reinventing the wheel.
Bad Example:
def is_even(num):
return num % 2 == 0
Good Example:
# Using Python's built-in function
is_even = lambda num: num % 2 == 0
The good example uses a lambda function, which is more concise. In general, prefer built-in Python functions like map()
, filter()
, and reduce()
when applicable.
5.6. Handle Errors Gracefully with Exceptions
Python encourages the use of exceptions for error handling rather than relying on error codes or complex condition checks. Proper exception handling ensures your code is robust and easier to debug.
Bad Example:
if x > 0:
result = 10 / x
else:
result = 0
Good Example:
try:
result = 10 / x
except ZeroDivisionError:
result = 0
By using exceptions, the code is more readable and easier to maintain.
5.7. Utilize Python’s Dynamic Typing Efficiently
Python's dynamic typing allows you to write code more flexibly, but it’s important to strike the right balance. Avoid overly complex type-checking, and rely on Python's duck typing principle: "If it looks like a duck and quacks like a duck, it's a duck."
5.8. Keep Functions Small and Focused
Pythonic functions should be small and do one thing well. Break down large functions into smaller, focused functions that can be reused in different parts of your program.
Bad Example:
def process_data(data):
# process data
# more code...
Good Example:
def clean_data(data):
# clean data
return cleaned_data
def analyze_data(data):
# analyze data
return analysis
Breaking down your functions into smaller, more manageable pieces makes your code easier to maintain.
5.9. Leverage Pythonic Idioms
Python is known for its elegant idioms, such as the ternary operator for simple conditional expressions or the with
statement for context management. Use these idioms to make your code more Pythonic.
6. Optimizing Performance in Python
While Python emphasizes simplicity and readability, it also provides tools for optimizing performance. Some tips to consider when optimizing Python code include:
Use built-in functions: Built-in Python functions are often more optimized than custom implementations.
Profile your code: Use Python’s
cProfile
module to identify performance bottlenecks.Leverage multi-threading or multiprocessing: For CPU-bound tasks, consider using the
multiprocessing
module.Use third-party libraries: Libraries like NumPy can significantly speed up computations involving large data.
Optimizing performance doesn’t mean sacrificing readability, but sometimes performance considerations are necessary for large-scale applications.
7. Tools to Help Write Pythonic Code
Several tools can help you write Pythonic code and adhere to best practices:
Linters: Tools like
flake8
andpylint
check your code for PEP 8 compliance and other coding issues.Formatters: Tools like
black
automatically format your code to meet PEP 8 standards.Static Type Checkers: Use
mypy
to perform static type checking and catch potential issues early.
These tools help ensure that your code remains clean, consistent, and Pythonic.
8. Conclusion: Developing Pythonic Habits for 2025
As Python continues to evolve in 2025, writing clean and Pythonic code will remain a vital skill for developers. By following best practices like adhering to PEP 8, using Python’s built-in features, and embracing Pythonic idioms, you can write code that is efficient, readable, and maintainable.
Remember, writing Pythonic code is a journey, not a destination. The more you practice and integrate these practices into your development routine, the more natural they will become. Strive to make Python your language of choice by writing code that is clean, elegant, and easy to maintain—after all, simplicity is the ultimate sophistication.
Happy coding!