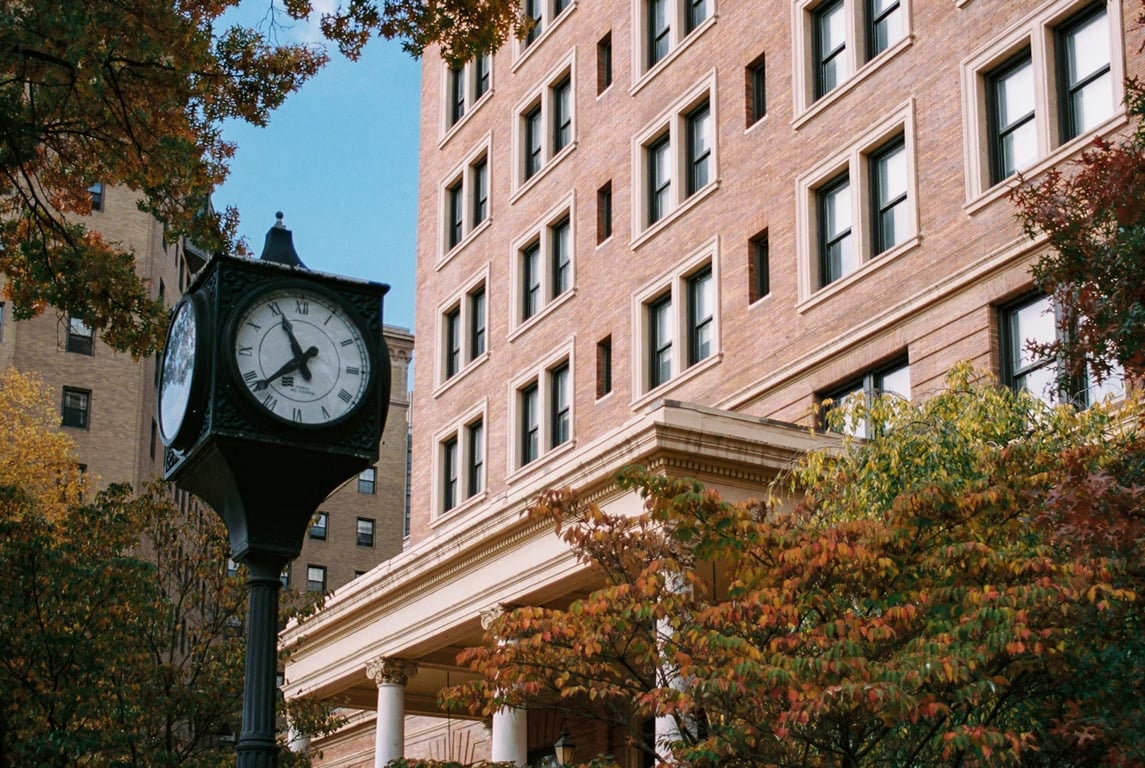
In Python, memory management is a critical aspect of programming that ensures efficient use of resources, particularly when handling large amounts of data or running long-running applications. Memory management in Python is largely automatic, but as a developer, it’s essential to understand how Python handles memory, especially when it comes to object deletion and garbage collection.
In this blog post, we will explore Python's memory management system, focusing on two key components: the del
statement and the garbage collection mechanism. We’ll explain how they work, provide examples, and discuss best practices for efficient memory usage in Python.
Table of Contents
Introduction to Python Memory Management
What Happens When an Object is Created?
The Role of the
del
StatementUnderstanding Python’s Garbage Collection
The Reference Counting Mechanism
Cyclic Garbage Collection
How to Optimize Memory Management in Python
Best Practices for Managing Memory in Python
Conclusion
1. Introduction to Python Memory Management
Memory management in Python refers to the process of allocating, managing, and freeing memory that is used by Python objects during the execution of a program. The Python memory manager automatically handles memory allocation and deallocation using two primary techniques: reference counting and garbage collection.
Reference counting is the most straightforward approach, where each object in memory keeps track of how many references point to it. When an object’s reference count drops to zero (i.e., no references to the object remain), the memory occupied by the object is released.
However, reference counting has its limitations, particularly when it comes to circular references, where objects reference each other in a cycle. To address this issue, Python uses a more sophisticated garbage collection mechanism that handles cyclic dependencies.
2. What Happens When an Object is Created?
Before diving into the specifics of del
and garbage collection, it’s important to understand what happens when an object is created in Python.
Object Creation and Reference Counting
When you create an object in Python, the following steps occur:
Memory Allocation: Python allocates memory for the new object.
Reference Count Incrementation: Python assigns a reference count to the object. The reference count is initially set to 1 when the object is created.
Here’s an example:
x = [1, 2, 3] # Create a new list object
In this example, a new list object [1, 2, 3]
is created, and the reference count for this object is incremented to 1. The variable x
holds a reference to this list.
3. The Role of the del
Statement
In Python, the del
statement is used to delete references to an object. This does not necessarily mean that the object is immediately removed from memory, but it decreases the reference count of the object by 1.
How del
Works
When you use the del
statement, Python reduces the reference count of the object associated with the variable. If the reference count drops to zero, the memory occupied by the object is released.
Here’s an example to demonstrate how del
works:
x = [1, 2, 3] # Create a list
y = x # y now references the same list as x
del x # Delete the reference to the list from x
print(y) # y still holds the reference to the list
n the example above:
The list
[1, 2, 3]
is created and assigned to the variablex
.The variable
y
is then assigned the reference to the same list.After using
del x
, the reference count for the list object decreases by 1. However, the object is not immediately destroyed becausey
still holds a reference to it.Finally, the program prints the list via
y
.
Important Note about del
The del
statement only removes the reference to the object. If the object is still referenced elsewhere, it will not be deleted from memory. Python’s garbage collection will take care of it later if no references remain.
4. Understanding Python’s Garbage Collection
The Reference Counting Mechanism
As mentioned earlier, Python uses reference counting as its primary memory management mechanism. Each object in Python has an associated reference count that tracks how many variables or objects refer to that object. When the reference count of an object becomes zero, it means that the object is no longer in use and its memory can be freed.
Let’s look at an example:
x = [1, 2, 3] # Reference count = 1
y = x # Reference count = 2
del x # Reference count = 1
del y # Reference count = 0, memory freed
In the above code, after the second del
, the reference count becomes zero, and the memory is freed. Python’s memory manager automatically handles the freeing of memory when the reference count drops to zero.
Cyclic Garbage Collection
Reference counting is effective for most scenarios, but it has limitations when it comes to cyclic references. Cyclic references occur when two or more objects reference each other, creating a cycle. In such cases, the reference count never drops to zero, even though the objects are no longer accessible by the program.
To handle cyclic references, Python uses cyclic garbage collection, which is a more sophisticated technique to identify and collect objects involved in circular references. The garbage collector runs periodically in the background to detect these cycles and free the memory associated with them.
Here’s an example of a cyclic reference:
class A:
def __init__(self):
self.b = None
class B:
def __init__(self):
self.a = None
# Creating a cyclic reference
a = A()
b = B()
a.b = b
b.a = a
In this example, a
references b
, and b
references a
, creating a cycle. Without cyclic garbage collection, these objects would never be deleted, even though they are no longer accessible in the program. Python’s garbage collector can detect this cycle and free the memory.
The gc
Module
Python provides the gc
(garbage collection) module to interact with the garbage collector. You can use this module to manually trigger garbage collection, control its behavior, and inspect the objects being tracked by the garbage collector.
For example:
import gc
# Enable automatic garbage collection
gc.enable()
# Manually trigger garbage collection
gc.collect()
5. How to Optimize Memory Management in Python
While Python’s memory management system is largely automatic, there are several best practices and techniques that can help you optimize memory usage in your programs.
1. Avoid Unnecessary References
Make sure to remove unnecessary references to objects to allow them to be garbage collected. Using del
can help clear references, but also be mindful of reference cycles and ensure that objects are not referenced by mistake.
2. Use Object Pools and Caching
In some cases, reusing objects rather than creating new ones can help optimize memory usage. Python provides tools like the functools.lru_cache()
decorator for caching the results of expensive function calls.
3. Utilize Memory-Optimized Data Structures
Consider using memory-optimized data structures like collections.namedtuple
or array.array
instead of standard lists or dictionaries for specific types of data.
4. Profiling Memory Usage
You can use the sys.getsizeof()
function to check the memory usage of Python objects. This can help identify areas in your code that use excessive memory.
Example:
import sys
my_list = [1, 2, 3]
print(sys.getsizeof(my_list)) # Output: memory size of my_list in bytes
5. Avoid Circular References
Circular references can complicate garbage collection. While Python’s garbage collector can handle cyclic references, it’s still a good idea to avoid them whenever possible.
6. Best Practices for Managing Memory in Python
Minimize global variables: Global variables tend to persist for the entire duration of the program, increasing memory usage.
Use context managers: For managing resources like file handles, using context managers (
with
statement) ensures that resources are released when no longer needed.Profile your code: Use tools like
memory_profiler
andobjgraph
to profile and visualize memory usage.Prefer built-in data structures: Python’s built-in data structures like
list
,tuple
, anddict
are highly optimized and generally more memory-efficient than custom data structures.
7. Conclusion
Memory management is a critical aspect of programming, especially in languages like Python, where automatic memory management is handled through reference counting and garbage collection. By understanding how Python’s memory management works—especially with tools like del
and the garbage collector—you can write more efficient programs and avoid common pitfalls such as memory leaks and excessive memory usage.
While Python’s garbage collection system automatically handles most memory management tasks, as a developer, you can optimize memory usage by being mindful of object references, avoiding circular references, and using memory-efficient data structures. With these best practices in mind, you’ll be able to write Python programs that perform well even with large datasets or in resource-constrained environments.
By taking control of memory management, you ensure your Python applications run efficiently and are capable of handling real-world workloads effectively.
Happy coding!