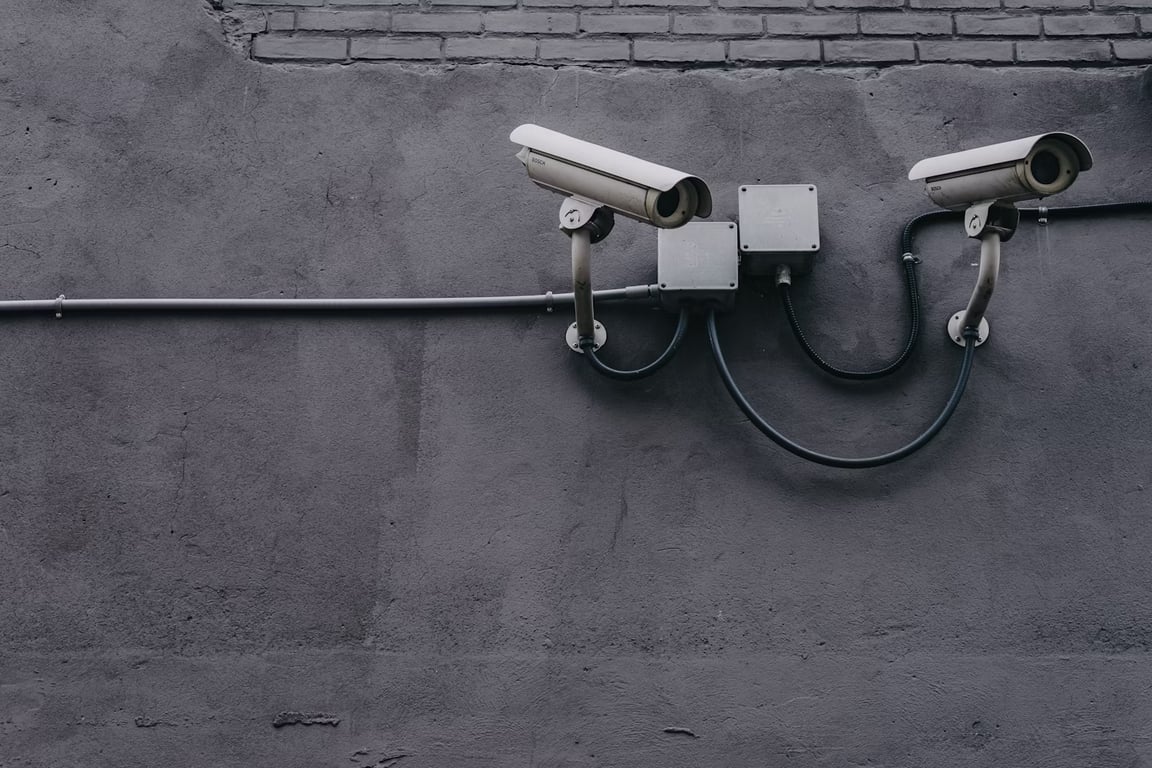
Photo by Scott Webb on Unsplash
Building secure web applications is a crucial part of any web development project. Security should be a priority from the beginning, as vulnerabilities in your application can be exploited by attackers, leading to data breaches, loss of trust, and even legal consequences. Python is one of the most popular programming languages for web development, with frameworks like Django and Flask used extensively to build web applications. In this blog, we'll explore common vulnerabilities in Python web applications and discuss best practices and techniques to secure them.
Table of Contents
Introduction to Web Application Security
Common Web Application Vulnerabilities
SQL Injection
Cross-Site Scripting (XSS)
Cross-Site Request Forgery (CSRF)
Insecure Deserialization
Sensitive Data Exposure
Securing Python Web Applications
Using Secure Frameworks
Validating User Input
Protecting Against SQL Injection
Preventing XSS Attacks
CSRF Protection
Managing User Authentication and Authorization
Encrypting Sensitive Data
Logging and Monitoring
Security Tools and Libraries for Python
Conclusion
1. Introduction to Web Application Security
Web application security involves protecting applications from threats that can compromise their integrity, confidentiality, and availability. It is essential to implement robust security measures to prevent unauthorized access, data manipulation, and data leaks. Developers need to be aware of the common vulnerabilities that attackers exploit and how to defend against them.
2. Common Web Application Vulnerabilities
SQL Injection
SQL injection is one of the most common and dangerous vulnerabilities in web applications. It occurs when an attacker manipulates SQL queries to execute arbitrary code on the database. This can lead to unauthorized access, data modification, or deletion.
Example:
def get_user_details(user_id):
query = f"SELECT * FROM users WHERE user_id = {user_id}"
result = db.execute(query)
return result
An attacker could pass in a value like 1 OR 1=1
to bypass authentication.
Use parameterized queries or ORM frameworks (like Django's ORM or SQLAlchemy) to safely handle user inputs.
Avoid concatenating user input directly into SQL queries.
Example using parameterized queries (Django ORM):
from django.contrib.auth.models import User
def get_user_details(user_id):
return User.objects.get(pk=user_id)
Cross-Site Scripting (XSS)
XSS attacks occur when an attacker injects malicious scripts into web pages viewed by other users. These scripts can execute on the client-side, potentially stealing cookies, session tokens, or other sensitive information.
Protection:Sanitize user inputs and escape HTML content before rendering it on the page.
Use libraries like HTMLPurifier or Django’s built-in template system, which automatically escapes content.
Example:
from django.utils.html import escape
def user_input_view(request):
user_input = request.GET.get('user_input', '')
sanitized_input = escape(user_input)
return HttpResponse(f'Your input was: {sanitized_input}')
Cross-Site Request Forgery (CSRF)
CSRF is an attack where an attacker tricks a user into making a request on a web application where they are authenticated, without the user's consent. This can lead to actions like changing account settings, making transactions, or deleting records.
Protection:Use anti-CSRF tokens that are tied to the user’s session.
Django includes built-in protection against CSRF attacks. For Flask, libraries like Flask-WTF can help.
Example in Django:
Make sure CSRF protection is enabled in your Django settings:
# settings.py
CSRF_COOKIE_SECURE = True
Then use the {% csrf_token %}
template tag in forms to include a CSRF token.
Insecure Deserialization
Insecure deserialization occurs when an attacker manipulates serialized data to inject malicious code. Deserializing unsafe data can lead to remote code execution, privilege escalation, or other attacks.
Protection:Avoid using insecure serialization methods like Python's
pickle
module for untrusted data.If you must use serialization, prefer safer formats like JSON or XML.
Sensitive Data Exposure
Sensitive data exposure refers to improper storage or transmission of sensitive information like passwords, credit card numbers, or personal information.
Protection:Always hash passwords before storing them using secure algorithms like bcrypt or Argon2.
Use TLS (HTTPS) for encrypting data in transit.
Avoid storing sensitive data unnecessarily and use tokenization or encryption for data at rest.
Example:
from django.contrib.auth.hashers import make_password
def store_password(password):
hashed_password = make_password(password)
# Store hashed_password in the database
3. Securing Python Web Applications
Now that we've identified some of the most common vulnerabilities, let's discuss how to secure Python web applications.
Using Secure Frameworks
Frameworks like Django and Flask come with built-in security features. Django, for example, has strong protection against SQL injection, XSS, and CSRF by default. Flask, while more lightweight, can also be secured with additional libraries.
Validating User Input
Always validate user input to ensure it conforms to the expected format. For example, if expecting an email address, ensure that the input matches the regular expression for a valid email. This reduces the chances of malicious input that could lead to vulnerabilities.
import re
def validate_email(email):
pattern = r"(^[a-z0-9]+[\._]?[a-z0-9]+[@]\w+[.]\w{2,3}$)"
if re.match(pattern, email):
return True
return False
Protecting Against SQL Injection
As mentioned, use parameterized queries to prevent SQL injection attacks. Many Python web frameworks provide built-in ORMs that automatically handle SQL queries securely.
For example, in Django, the ORM automatically escapes queries to prevent SQL injection.
from django.contrib.auth.models import User
def get_user_by_id(user_id):
return User.objects.get(pk=user_id)
In Flask with SQLAlchemy:
from flask_sqlalchemy import SQLAlchemy
db = SQLAlchemy()
def get_user_by_id(user_id):
user = db.session.query(User).filter(User.id == user_id).first()
return user
Preventing XSS Attacks
Ensure that user-provided data is sanitized before being rendered in your application. Use frameworks that automatically escape user inputs to avoid rendering malicious scripts.
In Django, template rendering automatically escapes content:
<p>{{ user_input }}</p>
This will escape any HTML tags or JavaScript in the user_input
variable.
CSRF Protection
In Django, CSRF protection is enabled by default. Ensure that the {% csrf_token %}
tag is included in your HTML forms. For Flask, use the Flask-WTF library to enable CSRF protection.
# Flask example with Flask-WTF
from flask_wtf import FlaskForm
from wtforms import StringField
class MyForm(FlaskForm):
name = StringField('Name')
Managing User Authentication and Authorization
Always use strong authentication methods like multi-factor authentication (MFA) where possible.
For Python web applications, use libraries like Django’s built-in authentication or Flask-Login for user session management.
Use JWT (JSON Web Tokens) or OAuth 2.0 for secure token-based authentication.
Encrypting Sensitive Data
Always encrypt sensitive data both in transit and at rest. Use TLS/SSL to encrypt data transmitted over the web. For data storage, use encryption libraries like PyCryptodome.
from Crypto.Cipher import AES
from Crypto.Util.Padding import pad, unpad
def encrypt_data(data, key):
cipher = AES.new(key, AES.MODE_CBC)
encrypted_data = cipher.encrypt(pad(data.encode(), AES.block_size))
return encrypted_data
4. Security Tools and Libraries for Python
There are several tools and libraries that can help enhance the security of your Python web applications:
Bandit: A security linter for Python that helps identify common security issues in your codebase.
Flask-Security: An extension for Flask that adds security features like user authentication, session management, and role-based access control.
Django Security Middleware: Django provides middleware to handle common security concerns like CSRF, XSS, and HTTP headers.
5. Conclusion
Securing Python web applications from common vulnerabilities is an ongoing process. By following best practices, such as using secure frameworks, validating input, preventing SQL injection, and encrypting sensitive data, you can significantly reduce the chances of a successful attack. Always stay up-to-date with security patches and consider using additional security tools to safeguard your web applications. Remember, a proactive approach to security is always better than dealing with the aftermath of a data breach.
By incorporating these security practices into your development workflow, you can build Python web applications that are not only functional but also secure.
Happy coding!