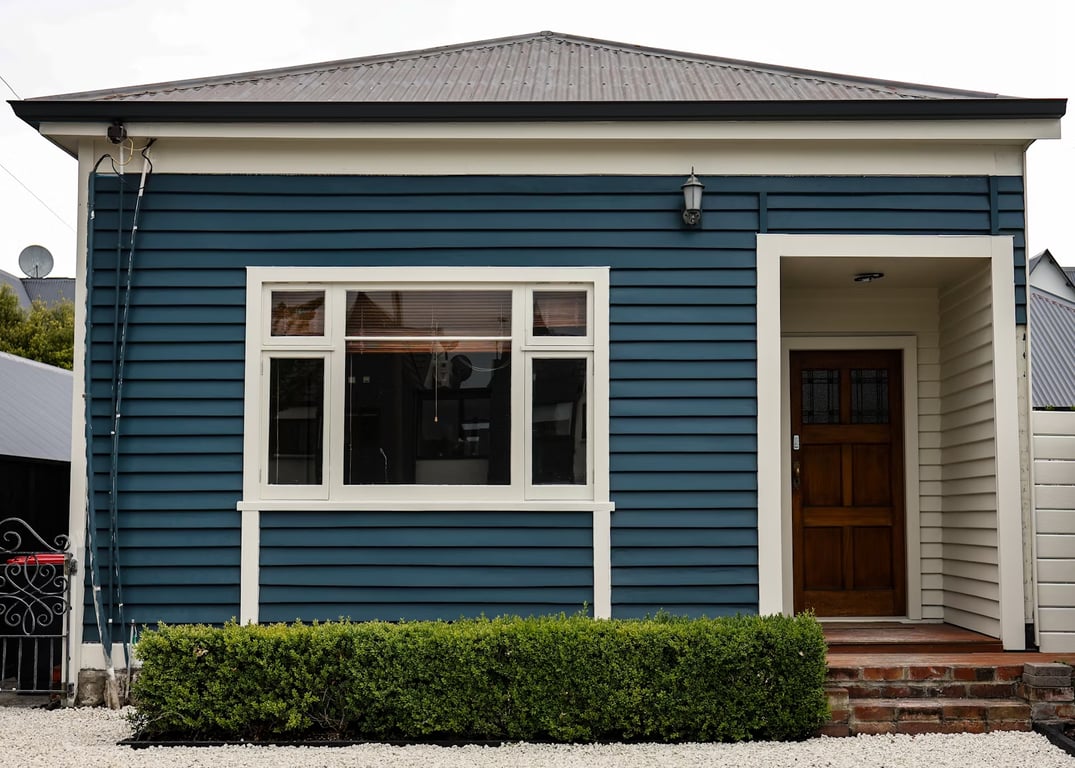
Photo by Diana Rafira on Unsplash
Building a blog website is a great way to get hands-on experience with web development, especially using Django, one of Python's most popular web frameworks. This step-by-step guide will walk you through the entire process of building a simple but functional blog website using Django. By the end of this tutorial, you will have learned how to set up Django, create models, views, templates, and forms, and handle user authentication.
Table of Contents
Prerequisites
Setting Up Your Django Project
Creating the Blog Model
Creating Views and Templates
Adding URLs
Handling User Authentication
Creating Blog Post Forms
Styling the Blog Website
Running the Blog Website Locally
Deploying the Blog Website
1. Prerequisites
Before starting, ensure that you have the following:
Python 3.x installed on your system.
Django installed. If not, you can install it via pip:
pip install django
A basic understanding of Python and web development concepts like HTTP requests, HTML, and CSS.
2. Setting Up Your Django Project
2.1. Create a New Django Project
First, create a directory for your project and navigate to it in your terminal.
mkdir myblog
cd myblog
Next, create a Django project by running the following command:
django-admin startproject myblog
This will generate a project directory with several files, including settings.py
, urls.py
, and wsgi.py
.
2.2. Create a Django App
In Django, an app is a web application that performs a specific task (like managing a blog). To create a blog app, run the following command:
python manage.py startapp blog
This creates a blog
directory with several files, including models.py
, views.py
, and urls.py
. You’ll define your blog-related code in this app.
2.3. Add the Blog App to the Project
Open the settings.py
file in the myblog
directory and add 'blog'
to the INSTALLED_APPS
list:
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'blog', # Add this line
]
3. Creating the Blog Model
The next step is to create the database model that will store the blog posts. Open the models.py
file in the blog
directory and define a Post
model:
from django.db import models
class Post(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
created_at = models.DateTimeField(auto_now_add=True)
updated_at = models.DateTimeField(auto_now=True)
def __str__(self):
return self.title
In this model:
title
stores the title of the blog post.content
stores the body of the post.created_at
andupdated_at
track the timestamps for when the post was created and last updated.
3.1. Apply Migrations
After defining the model, you need to create the database table. Run the following commands:
python manage.py makemigrations
python manage.py migrate
This will create the necessary database tables for your blog model.
4. Creating Views and Templates
4.1. Create Views for the Blog
In views.py
, you will create views to display the blog posts. Here’s an example of how to display all the blog posts:
from django.shortcuts import render
from .models import Post
def home(request):
posts = Post.objects.all() # Retrieve all posts
return render(request, 'blog/home.html', {'posts': posts})
This view fetches all the blog posts from the database and renders the home.html
template.
4.2. Create Templates
Create a templates
folder in the blog
directory, then create a home.html
file to display the posts:
<!DOCTYPE html>
<html>
<head>
<title>My Blog</title>
</head>
<body>
<h1>Welcome to My Blog</h1>
<ul>
{% for post in posts %}
<li>
<h2>{{ post.title }}</h2>
<p>{{ post.content|truncatewords:20 }}</p>
<p><a href="#">Read More</a></p>
</li>
{% endfor %}
</ul>
</body>
</html>
This template displays the title and a truncated version of the content for each blog post. You can click on "Read More" to view the full post.
5. Adding URLs
5.1. Define URLs for the Blog
Create a urls.py
file in the blog
directory and define a URL pattern for the homepage:
from django.urls import path
from . import views
urlpatterns = [
path('', views.home, name='home'),
]
5.2. Link Blog URLs to Project
In the main project’s urls.py
file (myblog/urls.py
), include the blog URLs:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('blog.urls')), # Include blog app URLs
]
6. Handling User Authentication
To allow users to register, log in, and log out, you can use Django's built-in authentication system.
6.1. Create Authentication Views
In the views.py
file of your blog
app, add views for login and logout:
from django.contrib.auth import login, logout
from django.shortcuts import render, redirect
from django.contrib.auth.forms import UserCreationForm
def user_register(request):
if request.method == 'POST':
form = UserCreationForm(request.POST)
if form.is_valid():
form.save()
return redirect('login')
else:
form = UserCreationForm()
return render(request, 'blog/register.html', {'form': form})
def user_login(request):
# Implement login logic here
pass
def user_logout(request):
logout(request)
return redirect('home')
6.2. Add Login and Logout Links in Templates
In the home.html
template, add links to log in or log out based on whether the user is authenticated:
{% if user.is_authenticated %}
<p>Hello, {{ user.username }}! <a href="{% url 'logout' %}">Logout</a></p>
{% else %}
<a href="{% url 'login' %}">Login</a> | <a href="{% url 'register' %}">Register</a>
{% endif %}
7. Creating Blog Post Forms
To allow users to create blog posts, you can create a form for submitting blog posts.
7.1. Define the Post Form
In forms.py
of the blog
app, create a form for adding new posts:
from django import forms
from .models import Post
class PostForm(forms.ModelForm):
class Meta:
model = Post
fields = ['title', 'content']
7.2. Create a View for Adding Posts
In views.py
, create a view to handle post creation:
from .forms import PostForm
def add_post(request):
if request.method == 'POST':
form = PostForm(request.POST)
if form.is_valid():
form.save()
return redirect('home')
else:
form = PostForm()
return render(request, 'blog/add_post.html', {'form': form})
7.3. Create a Template for the Post Form
Create a add_post.html
template to display the form:
<!DOCTYPE html>
<html>
<head>
<title>Add New Post</title>
</head>
<body>
<h1>Add a New Blog Post</h1>
<form method="POST">
{% csrf_token %}
{{ form.as_p }}
<button type="submit">Submit</button>
</form>
</body>
</html>
8. Styling the Blog Website
You can use CSS or frameworks like Bootstrap to style the blog website. Simply add a static
directory inside the blog
app and link your stylesheets to the templates.
9. Running the Blog Website Locally
To run the project locally and test it, use the following command:
python manage.py runserver
Visit http://127.0.0.1:8000/
in your browser to see your blog website.
10. Deploying the Blog Website
Once your blog website is ready and you’re happy with the functionality, it’s time to deploy it. You can deploy your Django app to platforms like Heroku, DigitalOcean, or AWS. You’ll need to set up a production database, configure the ALLOWED_HOSTS
, and collect static files.
Conclusion
Congratulations! You’ve now built a simple yet functional blog website using Django. This project serves as a foundation for learning web development concepts like models, views, templates, forms, and user authentication. You can continue to improve it by adding more features like comments, tags, categories, and pagination.
Happy coding!